mirror of
https://github.com/sigmasternchen/php-doc-en
synced 2025-03-29 23:38:56 +00:00
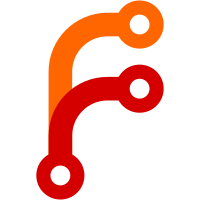
- Update all options to include the WriteConcern option - and refactored those options into entities git-svn-id: https://svn.php.net/repository/phpdoc/en/trunk@328543 c90b9560-bf6c-de11-be94-00142212c4b1
436 lines
9.2 KiB
XML
436 lines
9.2 KiB
XML
<?xml version="1.0" encoding="utf-8"?>
|
|
<!-- $Revision$ -->
|
|
|
|
<refentry xml:id="mongocollection.aggregate" xmlns="http://docbook.org/ns/docbook" xmlns:xlink="http://www.w3.org/1999/xlink">
|
|
<refnamediv>
|
|
<refname>MongoCollection::aggregate</refname>
|
|
<refpurpose>Perform an aggregation using the aggregation framework</refpurpose>
|
|
</refnamediv>
|
|
|
|
<refsect1 role="description">
|
|
&reftitle.description;
|
|
<methodsynopsis>
|
|
<modifier>public</modifier> <type>array</type><methodname>MongoCollection::aggregate</methodname>
|
|
<methodparam><type>array</type><parameter>pipeline</parameter></methodparam>
|
|
<methodparam choice="opt"><type>array</type><parameter>op</parameter></methodparam>
|
|
<methodparam choice="opt"><type>array</type><parameter>...</parameter></methodparam>
|
|
</methodsynopsis>
|
|
<para>
|
|
The MongoDB
|
|
<link xlink:href="&url.mongodb.docs.aggregation;">aggregation framework</link>
|
|
provides a means to calculate aggregated values without having to use
|
|
MapReduce. While MapReduce is powerful, it is often more difficult than
|
|
necessary for many simple aggregation tasks, such as totaling or averaging
|
|
field values.
|
|
</para>
|
|
<para>
|
|
This method accepts either a variable amount of pipeline operators, or a
|
|
single array of operators constituting the pipeline.
|
|
</para>
|
|
</refsect1>
|
|
|
|
<refsect1 role="parameters">
|
|
&reftitle.parameters;
|
|
<variablelist>
|
|
<varlistentry>
|
|
<term><parameter>pipeline</parameter></term>
|
|
<listitem>
|
|
<para>
|
|
An array of pipeline operators, or just the first operator.
|
|
</para>
|
|
</listitem>
|
|
</varlistentry>
|
|
<varlistentry>
|
|
<term><parameter>op</parameter></term>
|
|
<listitem>
|
|
<para>
|
|
The second pipeline operator.
|
|
</para>
|
|
</listitem>
|
|
</varlistentry>
|
|
<varlistentry>
|
|
<term><parameter>...</parameter></term>
|
|
<listitem>
|
|
<para>
|
|
Additional pipeline operators.
|
|
</para>
|
|
</listitem>
|
|
</varlistentry>
|
|
</variablelist>
|
|
</refsect1>
|
|
|
|
<refsect1 role="returnvalues">
|
|
&reftitle.returnvalues;
|
|
<para>
|
|
The result of the aggregation as an array. The <varname>ok</varname> will
|
|
be set to <literal>1</literal> on success, <literal>0</literal> on failure.
|
|
</para>
|
|
</refsect1>
|
|
|
|
<refsect1 role="errors">
|
|
&reftitle.errors;
|
|
<para>
|
|
When an error occurs an array with the following keys will be returned:
|
|
<itemizedlist>
|
|
<listitem>
|
|
<simpara>
|
|
<varname>errmsg</varname> - containing the reason for the failure
|
|
</simpara>
|
|
</listitem>
|
|
<listitem>
|
|
<simpara>
|
|
<varname>code</varname> - the errorcode of the failure
|
|
</simpara>
|
|
</listitem>
|
|
<listitem>
|
|
<simpara>
|
|
<varname>ok</varname> - will be set to 0.
|
|
</simpara>
|
|
</listitem>
|
|
</itemizedlist>
|
|
</para>
|
|
</refsect1>
|
|
|
|
<refsect1 role="examples">
|
|
&reftitle.examples;
|
|
<example xml:id="mongocollection.aggregate.example.basic">
|
|
<title><methodname>MongoCollection::aggregate</methodname> example</title>
|
|
<para>
|
|
The following example aggregation operation pivots data to create a set of
|
|
author names grouped by tags applied to an article. Call the aggregation
|
|
framework by issuing the following command:
|
|
</para>
|
|
<programlisting role="php">
|
|
<![CDATA[
|
|
<?php
|
|
$m = new Mongo;
|
|
$c = $m->selectDB("examples")->selectCollection("article");
|
|
$data = array (
|
|
'title' => 'this is my title',
|
|
'author' => 'bob',
|
|
'posted' => new MongoDate,
|
|
'pageViews' => 5,
|
|
'tags' => array ( 'fun', 'good', 'fun' ),
|
|
'comments' => array (
|
|
array (
|
|
'author' => 'joe',
|
|
'text' => 'this is cool',
|
|
),
|
|
array (
|
|
'author' => 'sam',
|
|
'text' => 'this is bad',
|
|
),
|
|
),
|
|
'other' =>array (
|
|
'foo' => 5,
|
|
),
|
|
);
|
|
$d = $c->insert($data, array("w" => 1));
|
|
|
|
$ops = array(
|
|
array(
|
|
'$project' => array(
|
|
"author" => 1,
|
|
"tags" => 1,
|
|
)
|
|
),
|
|
array('$unwind' => '$tags'),
|
|
array(
|
|
'$group' => array(
|
|
"_id" => array("tags" => '$tags'),
|
|
"authors" => array('$addToSet' => '$author'),
|
|
),
|
|
),
|
|
);
|
|
$results = $c->aggregate($ops);
|
|
var_dump($results);
|
|
?>
|
|
]]>
|
|
</programlisting>
|
|
&example.outputs;
|
|
<screen>
|
|
<![CDATA[
|
|
array(2) {
|
|
["result"]=>
|
|
array(2) {
|
|
[0]=>
|
|
array(2) {
|
|
["_id"]=>
|
|
array(1) {
|
|
["tags"]=>
|
|
string(4) "good"
|
|
}
|
|
["authors"]=>
|
|
array(1) {
|
|
[0]=>
|
|
string(3) "bob"
|
|
}
|
|
}
|
|
[1]=>
|
|
array(2) {
|
|
["_id"]=>
|
|
array(1) {
|
|
["tags"]=>
|
|
string(3) "fun"
|
|
}
|
|
["authors"]=>
|
|
array(1) {
|
|
[0]=>
|
|
string(3) "bob"
|
|
}
|
|
}
|
|
}
|
|
["ok"]=>
|
|
float(1)
|
|
}
|
|
]]>
|
|
</screen>
|
|
</example>
|
|
|
|
<para>
|
|
The following examples use the <link xlink:href="&url.mongodb.examples.zipcode;">zipcode data set</link>.
|
|
Use mongoimport to load this data set into your mongod instance.
|
|
</para>
|
|
|
|
<example xml:id="mongocollection.aggregate.example.zipcode.population">
|
|
<title><methodname>MongoCollection::aggregate</methodname> example</title>
|
|
<para>
|
|
To return all states with a population greater than 10 million, use the following aggregation operation:
|
|
</para>
|
|
<programlisting role="php">
|
|
<![CDATA[
|
|
<?php
|
|
$m = new Mongo;
|
|
$c = $m->selectDB("test")->selectCollection("zips");
|
|
|
|
$out = $c->aggregate(array(
|
|
'$group' => array(
|
|
'_id' => '$state',
|
|
'totalPop' => array('$sum' => '$pop')
|
|
)
|
|
),
|
|
array(
|
|
'$match' => array('totalPop' => array('$gte' => 10*1000*1000))
|
|
)
|
|
);
|
|
|
|
var_dump($out);
|
|
?>
|
|
]]>
|
|
</programlisting>
|
|
&example.outputs.similar;
|
|
<screen>
|
|
<![CDATA[
|
|
array(2) {
|
|
["result"]=>
|
|
array(7) {
|
|
[0]=>
|
|
array(2) {
|
|
["_id"]=>
|
|
string(2) "TX"
|
|
["totalPop"]=>
|
|
int(16986510)
|
|
}
|
|
[1]=>
|
|
array(2) {
|
|
["_id"]=>
|
|
string(2) "PA"
|
|
["totalPop"]=>
|
|
int(11881643)
|
|
}
|
|
[2]=>
|
|
array(2) {
|
|
["_id"]=>
|
|
string(2) "NY"
|
|
["totalPop"]=>
|
|
int(17990455)
|
|
}
|
|
[3]=>
|
|
array(2) {
|
|
["_id"]=>
|
|
string(2) "IL"
|
|
["totalPop"]=>
|
|
int(11430602)
|
|
}
|
|
[4]=>
|
|
array(2) {
|
|
["_id"]=>
|
|
string(2) "CA"
|
|
["totalPop"]=>
|
|
int(29760021)
|
|
}
|
|
[5]=>
|
|
array(2) {
|
|
["_id"]=>
|
|
string(2) "OH"
|
|
["totalPop"]=>
|
|
int(10847115)
|
|
}
|
|
[6]=>
|
|
array(2) {
|
|
["_id"]=>
|
|
string(2) "FL"
|
|
["totalPop"]=>
|
|
int(12937926)
|
|
}
|
|
}
|
|
["ok"]=>
|
|
float(1)
|
|
}
|
|
]]>
|
|
</screen>
|
|
</example>
|
|
|
|
<example xml:id="mongocollection.aggregate.example.zipcode.population.average">
|
|
<title><methodname>MongoCollection::aggregate</methodname> example</title>
|
|
<para>
|
|
To return the average populations for cities in each state, use the following aggregation operation:
|
|
</para>
|
|
<programlisting role="php">
|
|
<![CDATA[
|
|
<?php
|
|
$m = new Mongo;
|
|
$c = $m->selectDB("test")->selectCollection("zips");
|
|
|
|
$out = $c->aggregate(
|
|
array(
|
|
'$group' => array(
|
|
'_id' => array('state' => '$state', 'city' => '$city' ),
|
|
'pop' => array('$sum' => '$pop' )
|
|
)
|
|
),
|
|
array(
|
|
'$group' => array(
|
|
'_id' => '$_id.state',
|
|
'avgCityPop' => array('$avg' => '$pop')
|
|
)
|
|
)
|
|
);
|
|
|
|
var_dump($out);
|
|
|
|
]]>
|
|
</programlisting>
|
|
&example.outputs.similar;
|
|
<screen>
|
|
<![CDATA[
|
|
array(2) {
|
|
["result"]=>
|
|
array(51) {
|
|
[0]=>
|
|
array(2) {
|
|
["_id"]=>
|
|
string(2) "DC"
|
|
["avgCityPop"]=>
|
|
float(303450)
|
|
}
|
|
[1]=>
|
|
array(2) {
|
|
["_id"]=>
|
|
string(2) "DE"
|
|
["avgCityPop"]=>
|
|
float(14481.913043478)
|
|
}
|
|
[2]=>
|
|
array(2) {
|
|
["_id"]=>
|
|
string(2) "RI"
|
|
["avgCityPop"]=>
|
|
float(18933.283018868)
|
|
}
|
|
[3]=>
|
|
array(2) {
|
|
["_id"]=>
|
|
string(2) "AL"
|
|
["avgCityPop"]=>
|
|
float(7907.2152641879)
|
|
}
|
|
[4]=>
|
|
array(2) {
|
|
["_id"]=>
|
|
string(2) "NH"
|
|
["avgCityPop"]=>
|
|
float(5232.320754717)
|
|
}
|
|
...
|
|
[45]=>
|
|
array(2) {
|
|
["_id"]=>
|
|
string(2) "WY"
|
|
["avgCityPop"]=>
|
|
float(3359.9111111111)
|
|
}
|
|
[46]=>
|
|
array(2) {
|
|
["_id"]=>
|
|
string(2) "MN"
|
|
["avgCityPop"]=>
|
|
float(5335.4865853659)
|
|
}
|
|
[47]=>
|
|
array(2) {
|
|
["_id"]=>
|
|
string(2) "OK"
|
|
["avgCityPop"]=>
|
|
float(6155.7436399217)
|
|
}
|
|
[48]=>
|
|
array(2) {
|
|
["_id"]=>
|
|
string(2) "IL"
|
|
["avgCityPop"]=>
|
|
float(9931.0182450043)
|
|
}
|
|
[49]=>
|
|
array(2) {
|
|
["_id"]=>
|
|
string(2) "WI"
|
|
["avgCityPop"]=>
|
|
float(7323.0074850299)
|
|
}
|
|
[50]=>
|
|
array(2) {
|
|
["_id"]=>
|
|
string(2) "WV"
|
|
["avgCityPop"]=>
|
|
float(2759.1953846154)
|
|
}
|
|
}
|
|
["ok"]=>
|
|
float(1)
|
|
}
|
|
]]>
|
|
</screen>
|
|
</example>
|
|
|
|
</refsect1>
|
|
|
|
<refsect1 role="seealso">
|
|
&reftitle.seealso;
|
|
<simplelist>
|
|
<member>The MongoDB <link xlink:href="&url.mongodb.docs.aggregation;">aggregation framework</link></member>
|
|
</simplelist>
|
|
</refsect1>
|
|
|
|
</refentry>
|
|
|
|
<!-- Keep this comment at the end of the file
|
|
Local variables:
|
|
mode: sgml
|
|
sgml-omittag:t
|
|
sgml-shorttag:t
|
|
sgml-minimize-attributes:nil
|
|
sgml-always-quote-attributes:t
|
|
sgml-indent-step:1
|
|
sgml-indent-data:t
|
|
indent-tabs-mode:nil
|
|
sgml-parent-document:nil
|
|
sgml-default-dtd-file:"~/.phpdoc/manual.ced"
|
|
sgml-exposed-tags:nil
|
|
sgml-local-catalogs:nil
|
|
sgml-local-ecat-files:nil
|
|
End:
|
|
vim600: syn=xml fen fdm=syntax fdl=2 si
|
|
vim: et tw=78 syn=sgml
|
|
vi: ts=1 sw=1
|
|
-->
|