mirror of
https://github.com/sigmasternchen/php-doc-en
synced 2025-03-15 16:38:54 +00:00
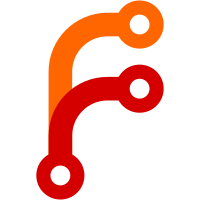
files again. (Only this time, the way it is divided up makes a bit of sense.) git-svn-id: https://svn.php.net/repository/phpdoc/en/trunk@9863 c90b9560-bf6c-de11-be94-00142212c4b1
385 lines
10 KiB
Text
385 lines
10 KiB
Text
<chapter id="language.operators">
|
|
<title>Operators</title>
|
|
<simpara>
|
|
</simpara>
|
|
|
|
<sect1 id="language.operators.arithmetic">
|
|
<title>Arithmetic Operators</title>
|
|
<simpara>
|
|
Remember basic arithmetic from school? These work just
|
|
like those.
|
|
</simpara>
|
|
|
|
<table>
|
|
<title>Arithmetic Operators</title>
|
|
<tgroup cols="3">
|
|
<thead>
|
|
<row>
|
|
<entry>example</entry>
|
|
<entry>name</entry>
|
|
<entry>result</entry>
|
|
</row>
|
|
</thead>
|
|
<tbody>
|
|
<row>
|
|
<entry>$a + $b</entry>
|
|
<entry>Addition</entry>
|
|
<entry>Sum of $a and $b.</entry>
|
|
</row>
|
|
<row>
|
|
<entry>$a - $b</entry>
|
|
<entry>Subtraction</entry>
|
|
<entry>Remainder of $b subtracted from $a.</entry>
|
|
</row>
|
|
<row>
|
|
<entry>$a * $b</entry>
|
|
<entry>Multiplication</entry>
|
|
<entry>Product of $a and $b.</entry>
|
|
</row>
|
|
<row>
|
|
<entry>$a / $b</entry>
|
|
<entry>Division</entry>
|
|
<entry>Dividend of $a and $b.</entry>
|
|
</row>
|
|
<row>
|
|
<entry>$a % $b</entry>
|
|
<entry>Modulus</entry>
|
|
<entry>Remainder of $a divided by $b.</entry>
|
|
</row>
|
|
</tbody>
|
|
</tgroup>
|
|
</table>
|
|
|
|
<!-- This appears to be inaccurate. Division always returns a float.
|
|
<simpara>
|
|
The division operator ("/") returns an integer value (the result
|
|
of an integer division) if the two operands are integers (or
|
|
strings that get converted to integers). If either operand is a
|
|
floating-point value, floating-point division is performed.
|
|
</simpara>
|
|
-->
|
|
|
|
<sect1 id="language.operators.string">
|
|
<title>String Operators</title>
|
|
<simpara>
|
|
There is only really one string operator -- the concatenation
|
|
operator (".").
|
|
</simpara>
|
|
<para>
|
|
<informalexample><programlisting>
|
|
$a = "Hello ";
|
|
$b = $a . "World!"; // now $b = "Hello World!"
|
|
</programlisting></informalexample>
|
|
</para>
|
|
|
|
<sect1 id="language.operators.assignment">
|
|
<title>Assignment Operators</title>
|
|
<simpara>
|
|
The basic assignment operator is "=". Your first inclination might
|
|
be to think of this as "equal to". Don't. It really means that
|
|
the the left operand gets set to the value of the expression on the
|
|
rights (that is, "gets set to").
|
|
</simpara>
|
|
<para>
|
|
The value of an assignment expression is the value assigned. That
|
|
is, the value of "$a = 3" is 3. This allows you to do some tricky
|
|
things: <informalexample><programlisting>
|
|
$a = ($b = 4) + 5; // $a is equal to 9 now, and $b has been set to 4.
|
|
</programlisting></informalexample>
|
|
</para>
|
|
<para>
|
|
In addition to the basic assignment operator, there are "combined
|
|
operators" for all of the binary arithmetic and string operators
|
|
that allow you to use a value in an expression and then set its
|
|
value to the result of that expression. For example: <informalexample><programlisting>
|
|
$a = 3;
|
|
$a += 5; // sets $a to 8, as if we had said: $a = $a + 5;
|
|
$b = "Hello ";
|
|
$b .= "There!"; // sets $b to "Hello There!", just like $b = $b . "There!";
|
|
</programlisting></informalexample>
|
|
</para>
|
|
|
|
<sect1 id="language.operators.bitwise">
|
|
<title>Bitwise Operators</title>
|
|
<simpara>
|
|
Bitwise operators allow you to turn specific bits within an integer
|
|
on or off.
|
|
</simpara>
|
|
|
|
<table>
|
|
<title>Bitwise Operators</title>
|
|
<tgroup cols="3">
|
|
<thead>
|
|
<row>
|
|
<entry>example</entry>
|
|
<entry>name</entry>
|
|
<entry>result</entry>
|
|
</row>
|
|
</thead>
|
|
<tbody>
|
|
<row>
|
|
<entry>$a & $b</entry>
|
|
<entry>And</entry>
|
|
<entry>Bits that are set in both $a and $b are set.</entry>
|
|
</row>
|
|
<row>
|
|
<entry>$a | $b</entry>
|
|
<entry>Or</entry>
|
|
<entry>Bits that are set in either $a or $b are set.</entry>
|
|
</row>
|
|
<row>
|
|
<entry>~ $a</entry>
|
|
<entry>Not</entry>
|
|
<entry>Bits that are set in $a are not set, and vice versa.</entry>
|
|
</row>
|
|
<row>
|
|
<entry>$a << $b</entry>
|
|
<entry>Shift left</entry>
|
|
<entry>Shift the bits of $a $b steps to the left (each step means "multiply by two")</entry>
|
|
</row>
|
|
<row>
|
|
<entry>$a >> $b</entry>
|
|
<entry>Shift right</entry>
|
|
<entry>Shift the bits of $a $b steps to the right (each step means "divide by two")</entry>
|
|
</row>
|
|
</tbody>
|
|
</tgroup>
|
|
</table>
|
|
|
|
<sect1 id="language.operators.logical">
|
|
<title>Logical Operators</title>
|
|
|
|
<table>
|
|
<title>Logical Operators</title>
|
|
<tgroup cols="3">
|
|
<thead>
|
|
<row>
|
|
<entry>example</entry>
|
|
<entry>name</entry>
|
|
<entry>result</entry>
|
|
</row>
|
|
</thead>
|
|
<tbody>
|
|
<row>
|
|
<entry>$a and $b</entry>
|
|
<entry>And</entry>
|
|
<entry>True of both $a and $b are true.</entry>
|
|
</row>
|
|
<row>
|
|
<entry>$a or $b</entry>
|
|
<entry>Or</entry>
|
|
<entry>True if either $a or $b is true.</entry>
|
|
</row>
|
|
<row>
|
|
<entry>$a xor $b</entry>
|
|
<entry>Or</entry>
|
|
<entry>True if either $a or $b is true, but not both.</entry>
|
|
</row>
|
|
<row>
|
|
<entry>! $a</entry>
|
|
<entry>Not</entry>
|
|
<entry>True if $a is not true.</entry>
|
|
</row>
|
|
<row>
|
|
<entry>$a && $b</entry>
|
|
<entry>And</entry>
|
|
<entry>True of both $a and $b are true.</entry>
|
|
</row>
|
|
<row>
|
|
<entry>$a || $b</entry>
|
|
<entry>Or</entry>
|
|
<entry>True if either $a or $b is true.</entry>
|
|
</row>
|
|
</tbody>
|
|
</tgroup>
|
|
</table>
|
|
|
|
<simpara>
|
|
The reason for the two different variations of "and" and "or"
|
|
operators is that they operate at different precedences. (See below.)
|
|
</simpara>
|
|
|
|
<sect1 id="language.operators.comparison">
|
|
<title>Comparison Operators</title>
|
|
<simpara>
|
|
Comparison operators, as their name imply, allow you to compare two
|
|
values.
|
|
</simpara>
|
|
|
|
<table>
|
|
<title>Comparson Operators</title>
|
|
<tgroup cols="3">
|
|
<thead>
|
|
<row>
|
|
<entry>example</entry>
|
|
<entry>name</entry>
|
|
<entry>result</entry>
|
|
</row>
|
|
</thead>
|
|
<tbody>
|
|
<row>
|
|
<entry>$a == $b</entry>
|
|
<entry>Equal</entry>
|
|
<entry>True if $a is equal to $b.</entry>
|
|
</row>
|
|
<row>
|
|
<entry>$a != $b</entry>
|
|
<entry>Not equal</entry>
|
|
<entry>True if $a is not equal to $b.</entry>
|
|
</row>
|
|
<row>
|
|
<entry>$a < $b</entry>
|
|
<entry>Less than</entry>
|
|
<entry>True if $a is strictly less than $b.</entry>
|
|
</row>
|
|
<row>
|
|
<entry>$a > $b</entry>
|
|
<entry>Greater than</entry>
|
|
<entry>True if $a is strictly greater than $b.</entry>
|
|
</row>
|
|
<row>
|
|
<entry>$a <= $b</entry>
|
|
<entry>Less than or equal to </entry>
|
|
<entry>True if $a is less than or equal to $b.</entry>
|
|
</row>
|
|
<row>
|
|
<entry>$a >= $b</entry>
|
|
<entry>Greater than or equal to </entry>
|
|
<entry>True if $a is greater than or equal to $b.</entry>
|
|
</row>
|
|
</tbody>
|
|
</tgroup>
|
|
</table>
|
|
|
|
<para>
|
|
Another conditional operator is the "?:" (or trinary) operator, which
|
|
operates as in C and many other languages.
|
|
<informalexample><programlisting>(expr1) ? (expr2) : (expr3);</programlisting></informalexample>
|
|
This expression returns to <replaceable>expr2</replaceable> if
|
|
<replaceable>expr1</replaceable> evalutes to true, and expr3 if
|
|
<replaceable>expr1</replaceable> evaluates to false.
|
|
|
|
<sect1 id="language.operators.precedence">
|
|
<title>Operator Precedence</title>
|
|
<para>
|
|
The precedence of an operator specifies how "tightly" it binds
|
|
two expressions together. For example, in the expression
|
|
<literal>1 + 5 * 3</literal>, the answer is 16 and not
|
|
18 because the multiplication ("*") operator has a higher precedence
|
|
than the addition ("+") operator.
|
|
<para>
|
|
The following table lists the precedence of operators with the
|
|
lowest-precedence operators listed first.
|
|
|
|
<table>
|
|
<title>Operator Precedence</title>
|
|
<tgroup cols="2">
|
|
<thead>
|
|
<row>
|
|
<entry>Associativity</entry>
|
|
<entry>Operators</entry>
|
|
</row>
|
|
</thead>
|
|
<tbody>
|
|
<row>
|
|
<entry>left</entry>
|
|
<entry>,</entry>
|
|
</row>
|
|
<row>
|
|
<entry>left</entry>
|
|
<entry>or</entry>
|
|
</row>
|
|
<row>
|
|
<entry>left</entry>
|
|
<entry>xor</entry>
|
|
</row>
|
|
<row>
|
|
<entry>left</entry>
|
|
<entry>and</entry>
|
|
</row>
|
|
<row>
|
|
<entry>right</entry>
|
|
<entry>print</entry>
|
|
</row>
|
|
<row>
|
|
<entry>left</entry>
|
|
<entry>= += -= *= /= .= %= &= != ~= <<= >>=</entry>
|
|
</row>
|
|
<row>
|
|
<entry>left</entry>
|
|
<entry>? :</entry>
|
|
</row>
|
|
<row>
|
|
<entry>left</entry>
|
|
<entry>||</entry>
|
|
</row>
|
|
<row>
|
|
<entry>left</entry>
|
|
<entry>&&</entry>
|
|
</row>
|
|
<row>
|
|
<entry>left</entry>
|
|
<entry>|</entry>
|
|
</row>
|
|
<row>
|
|
<entry>left</entry>
|
|
<entry>^</entry>
|
|
</row>
|
|
<row>
|
|
<entry>left</entry>
|
|
<entry>&</entry>
|
|
</row>
|
|
<row>
|
|
<entry>non-associative</entry>
|
|
<entry>== !=</entry>
|
|
</row>
|
|
<row>
|
|
<entry>non-associative</entry>
|
|
<entry>< <= > >=</entry>
|
|
</row>
|
|
<row>
|
|
<entry>left</entry>
|
|
<entry><< >></entry>
|
|
</row>
|
|
<row>
|
|
<entry>left</entry>
|
|
<entry>+ - .</entry>
|
|
</row>
|
|
<row>
|
|
<entry>left</entry>
|
|
<entry>* / %</entry>
|
|
</row>
|
|
<row>
|
|
<entry>right</entry>
|
|
<entry>! ~ ++ -- (int) (double) (string) (array) (object) @</entry>
|
|
</row>
|
|
<row>
|
|
<entry>right</entry>
|
|
<entry>[</entry>
|
|
</row>
|
|
<row>
|
|
<entry>non-associative</entry>
|
|
<entry>new</entry>
|
|
</row>
|
|
</tbody>
|
|
</tgroup>
|
|
</table>
|
|
|
|
</chapter>
|
|
|
|
<!-- Keep this comment at the end of the file
|
|
Local variables:
|
|
mode: sgml
|
|
sgml-omittag:t
|
|
sgml-shorttag:t
|
|
sgml-minimize-attributes:nil
|
|
sgml-always-quote-attributes:t
|
|
sgml-indent-step:1
|
|
sgml-indent-data:t
|
|
sgml-parent-document:nil
|
|
sgml-default-dtd-file:"../manual.ced"
|
|
sgml-exposed-tags:nil
|
|
sgml-local-catalogs:nil
|
|
sgml-local-ecat-files:nil
|
|
End:
|
|
-->
|