mirror of
https://github.com/sigmasternchen/php-doc-en
synced 2025-03-15 16:38:54 +00:00
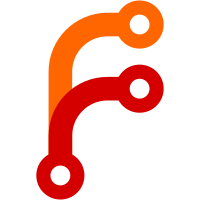
git-svn-id: https://svn.php.net/repository/phpdoc/en/trunk@10617 c90b9560-bf6c-de11-be94-00142212c4b1
1232 lines
38 KiB
Text
1232 lines
38 KiB
Text
|
|
<reference id="ref.oci8">
|
|
<title>Oracle 8 functions</title>
|
|
<titleabbrev>OCI8</titleabbrev>
|
|
<partintro>
|
|
<simpara>
|
|
These functions allow you to access Oracle8 and Oracle7 databases. It
|
|
uses the Oracle8 Call-Interface (OCI8). You will need the Oracle8 client
|
|
libraries to use this extension.
|
|
<simpara>
|
|
This extension is more flexible than the standard Oracle
|
|
extension. It supports binding of global and local PHP variables
|
|
to Oracle placeholders, has full LOB, FILE and ROWID support
|
|
and allows you to use user-supplied define variables.
|
|
</partintro>
|
|
|
|
<!--
|
|
OCIBindByName
|
|
OCIDefineByName
|
|
OCIColumnIsNULL
|
|
OCIColumnName
|
|
OCIColumnSize
|
|
OCIColumnType
|
|
OCIExecute
|
|
OCIFetch
|
|
OCIFetchInto
|
|
OCIFetchStatement
|
|
OCIInternalDebug
|
|
OCILogoff
|
|
OCILogon
|
|
OCIPLogon
|
|
OCINLogon
|
|
OCIError
|
|
OCICommit
|
|
OCIRollback
|
|
OCINewCursor
|
|
OCINewDescriptor
|
|
OCIRowCount
|
|
OCINumCols
|
|
OCIParse
|
|
OCIResult
|
|
OCIServerVersion
|
|
OCIStatementType
|
|
OCIFreeStatement
|
|
OCIFreeCursor
|
|
-->
|
|
|
|
<refentry id="function.ocidefinebyname">
|
|
<refnamediv>
|
|
<refname>OCIDefineByName</refname>
|
|
<refpurpose>Use a PHP variable for the define-step during a SELECT</refpurpose>
|
|
</refnamediv>
|
|
<refsect1>
|
|
<title>Description</title>
|
|
<funcsynopsis>
|
|
<funcdef>int <function>OCIDefineByName</function></funcdef>
|
|
<paramdef>int <parameter>stmt</parameter></paramdef>
|
|
<paramdef>string <parameter>Column-Name</parameter></paramdef>
|
|
<paramdef>mixed &<parameter>variable</parameter></paramdef>
|
|
<paramdef>int <parameter><optional>type</optional></parameter></paramdef>
|
|
</funcsynopsis>
|
|
<para>
|
|
<function>OCIDefineByName</function> uses fetches SQL-Columns
|
|
into user-defined PHP-Variables. Be careful that Oracle user
|
|
ALL-UPPERCASE column-names, whereby in your select you can also
|
|
write lower-case. <function>OCIDefineByName</function> expects
|
|
the <parameter>Column-Name</parameter> to be in uppercase. If you
|
|
define a variable that doesn't exists in you select statement, no
|
|
error will be given!
|
|
</para>
|
|
<para>
|
|
If you need to define an abstract Datatype (LOB/ROWID/BFILE) you
|
|
need to allocate it first using
|
|
<function>OCINewDescriptor</function> function. See also the
|
|
<function>OCIBindByName</function> function.
|
|
</para>
|
|
<example>
|
|
<title>OCIDefineByName</title>
|
|
<programlisting>
|
|
<?php
|
|
/* OCIDefineByPos example thies@digicol.de (980219) */
|
|
|
|
$conn = OCILogon("scott","tiger");
|
|
|
|
$stmt = OCIParse($conn,"select empno, ename from emp");
|
|
|
|
/* the define MUST be done BEFORE ociexecute! */
|
|
|
|
OCIDefineByName($stmt,"EMPNO",&$empno);
|
|
OCIDefineByName($stmt,"ENAME",&$ename);
|
|
|
|
OCIExecute($stmt);
|
|
|
|
while (OCIFetch($stmt)) {
|
|
echo "empno:".$empno."\n";
|
|
echo "ename:".$ename."\n";
|
|
}
|
|
|
|
OCIFreeStatement($stmt);
|
|
OCILogoff($conn);
|
|
?></programlisting></example>
|
|
|
|
</refsect1>
|
|
</refentry>
|
|
|
|
<refentry id="function.ocibindbyname">
|
|
<refnamediv>
|
|
<refname>OCIBindByName</refname>
|
|
<refpurpose>Bind a PHP variable to an Oracle Placeholder</refpurpose>
|
|
</refnamediv>
|
|
<refsect1>
|
|
<title>Description</title>
|
|
<funcsynopsis>
|
|
<funcdef>int <function>OCIBindByName</function></funcdef>
|
|
<paramdef>int <parameter>stmt</parameter></paramdef>
|
|
<paramdef>string <parameter>ph_name</parameter></paramdef>
|
|
<paramdef>mixed &<parameter>variable</parameter></paramdef>
|
|
<paramdef>int<parameter>length</parameter></paramdef>
|
|
<paramdef>int <parameter><optional>type</optional></parameter></paramdef>
|
|
</funcsynopsis>
|
|
<para>
|
|
<function>OCIBindByName</function> binds the PHP variable
|
|
<parameter>variable</parameter> to the Oracle placeholder
|
|
<parameter>ph_name</parameter>. Whether it will be used for
|
|
input or output will be determined run-time, and the necessary
|
|
storage space will be allocated. The
|
|
<parameter>length</parameter> paramter sets the maximum length
|
|
for the bind. If you set <parameter>length</parameter> to -1
|
|
<function>OCIBindByName</function> will use the current length of
|
|
<parameter>variable</parameter> to set the maximum length.
|
|
</para>
|
|
<para>
|
|
If you need to bind an abstract Datatype (LOB/ROWID/BFILE) you
|
|
need to allocate it first using
|
|
<function>OCINewDescriptor</function> function. The
|
|
<parameter>length</parameter> is not used for abstract Datatypes
|
|
and should be set to -1. The <parameter>type</parameter> variable
|
|
tells oracle, what kind of descriptor we want to use. Possible
|
|
values are: OCI_B_FILE (Binary-File), OCI_B_CFILE
|
|
(Character-File), OCI_B_CLOB (Character-LOB), OCI_B_BLOB
|
|
(Binary-LOB) and OCI_B_ROWID (ROWID).
|
|
</para>
|
|
<example>
|
|
<title>OCIDefineByName</title>
|
|
<programlisting>
|
|
<?php
|
|
/* OCIBindByPos example thies@digicol.de (980221)
|
|
|
|
inserts 3 resords into emp, and uses the ROWID for updating the
|
|
records just after the insert.
|
|
*/
|
|
|
|
$conn = OCILogon("scott","tiger");
|
|
|
|
$stmt = OCIParse($conn,"insert into emp (empno, ename) ".
|
|
"values (:empno,:ename) ".
|
|
"returning ROWID into :rid");
|
|
|
|
$data = array(1111 => "Larry", 2222 => "Bill", 3333 => "Jim");
|
|
|
|
$rowid = OCINewDescriptor($conn,OCI_D_ROWID);
|
|
|
|
OCIBindByName($stmt,":empno",&$empno,32);
|
|
OCIBindByName($stmt,":ename",&$ename,32);
|
|
OCIBindByName($stmt,":rid",&$rowid,-1,OCI_B_ROWID);
|
|
|
|
$update = OCIParse($conn,"update emp set sal = :sal where ROWID = :rid");
|
|
OCIBindByName($update,":rid",&$rowid,-1,OCI_B_ROWID);
|
|
OCIBindByName($update,":sal",&$sal,32);
|
|
|
|
$sal = 10000;
|
|
|
|
while (list($empno,$ename) = each($data)) {
|
|
OCIExecute($stmt);
|
|
OCIExecute($update);
|
|
}
|
|
|
|
$rowid->free();
|
|
|
|
OCIFreeStatement($update);
|
|
OCIFreeStatement($stmt);
|
|
|
|
$stmt = OCIParse($conn,"select * from emp where empno in (1111,2222,3333)");
|
|
OCIExecute($stmt);
|
|
while (OCIFetchInto($stmt,&$arr,OCI_ASSOC)) {
|
|
var_dump($arr);
|
|
}
|
|
OCIFreeStatement($stmt);
|
|
|
|
/* delete our "junk" from the emp table.... */
|
|
$stmt = OCIParse($conn,"delete from emp where empno in (1111,2222,3333)");
|
|
OCIExecute($stmt);
|
|
OCIFreeStatement($stmt);
|
|
|
|
OCILogoff($conn);
|
|
?></programlisting></example>
|
|
</refsect1>
|
|
</refentry>
|
|
|
|
<refentry id="function.ocilogon">
|
|
<refnamediv>
|
|
<refname>OCILogon</refname>
|
|
<refpurpose>Establishes a connection to Oracle</refpurpose>
|
|
</refnamediv>
|
|
<refsect1>
|
|
<title>Description</title>
|
|
<funcsynopsis>
|
|
<funcdef>int <function>OCILogon</function></funcdef>
|
|
<paramdef>string <parameter>username</parameter></paramdef>
|
|
<paramdef>string <parameter>password</parameter></paramdef>
|
|
<paramdef>string <parameter><optional>ORACLE_SID</optional></parameter></paramdef>
|
|
</funcsynopsis>
|
|
<para>
|
|
<function>OCILogon</function> returns an connection identified
|
|
needed for most other OCI calls. If the optional third parameter is not specified,
|
|
PHP uses the environment variable ORACLE_SID to determine which database to connect
|
|
to. Connections are shared at the page level when using <function>OCILogon</function>.
|
|
This means that commits and rollbacks apply to all open transactions in the page,
|
|
even if you have created multiple connections.
|
|
</para>
|
|
<para>
|
|
This example demonstrates how the connections are shared.
|
|
<example>
|
|
<title>OCILogon</title>
|
|
<programlisting>
|
|
<?php
|
|
print "<HTML><PRE>";
|
|
$db = "";
|
|
|
|
$c1 = ocilogon("scott","tiger",$db);
|
|
$c2 = ocilogon("scott","tiger",$db);
|
|
|
|
function create_table($conn)
|
|
{ $stmt = ociparse($conn,"create table scott.hallo (test
|
|
varchar2(32))");
|
|
ociexecute($stmt);
|
|
echo $conn." created table\n\n";
|
|
}
|
|
|
|
function drop_table($conn)
|
|
{ $stmt = ociparse($conn,"drop table scott.hallo");
|
|
ociexecute($stmt);
|
|
echo $conn." dropped table\n\n";
|
|
}
|
|
|
|
function insert_data($conn)
|
|
{ $stmt = ociparse($conn,"insert into scott.hallo values($conn || ' ' || to_char(sysdate,'DD-MON-YY HH24:MI:SS'))");
|
|
ociexecute($stmt,OCI_DEFAULT);
|
|
echo $conn." inserted hallo\n\n";
|
|
}
|
|
|
|
function delete_data($conn)
|
|
{ $stmt = ociparse($conn,"delete from scott.hallo");
|
|
ociexecute($stmt,OCI_DEFAULT);
|
|
echo $conn." deleted hallo\n\n";
|
|
}
|
|
|
|
function commit($conn)
|
|
{ ocicommit($conn);
|
|
echo $conn." commited\n\n";
|
|
}
|
|
|
|
function rollback($conn)
|
|
{ ocirollback($conn);
|
|
echo $conn." rollback\n\n";
|
|
}
|
|
|
|
function select_data($conn)
|
|
{ $stmt = ociparse($conn,"select * from scott.hallo");
|
|
ociexecute($stmt,OCI_DEFAULT);
|
|
echo $conn."----selecting\n\n";
|
|
while (ocifetch($stmt))
|
|
echo $conn." <".ociresult($stmt,"TEST").">\n\n";
|
|
echo $conn."----done\n\n";
|
|
}
|
|
|
|
create_table($c1);
|
|
insert_data($c1); // Insert a row using c1
|
|
insert_data($c2); // Insert a row using c2
|
|
|
|
select_data($c1); // Results of both inserts are returned
|
|
select_data($c2);
|
|
|
|
rollback($c1); // Rollback using c1
|
|
|
|
select_data($c1); // Both inserts have been rolled back
|
|
select_data($c2);
|
|
|
|
insert_data($c2); // Insert a row using c2
|
|
commit($c2); // commit using c2
|
|
|
|
select_data($c1); // result of c2 insert is returned
|
|
|
|
delete_data($c1); // delete all rows in table using c1
|
|
select_data($c1); // no rows returned
|
|
select_data($c2); // no rows returned
|
|
commit($c1); // commit using c1
|
|
|
|
select_data($c1); // no rows returned
|
|
select_data($c2); // no rows returned
|
|
|
|
|
|
drop_table($c1);
|
|
print "</PRE></HTML>";
|
|
?></programlisting></example>
|
|
<simpara>
|
|
See also <function>OCIPLogon</function> and
|
|
<function>OCINLogon</function>.
|
|
</refsect1>
|
|
</refentry>
|
|
|
|
<refentry id="function.ociplogon">
|
|
<refnamediv>
|
|
<refname>OCIPLogon</refname>
|
|
<refpurpose>Connect to an Oracle database and log on using a persistant connection.
|
|
Returns a new session.</refpurpose>
|
|
</refnamediv>
|
|
<refsect1>
|
|
<title>Description</title>
|
|
<funcsynopsis>
|
|
<funcdef>int <function>OCIPLogon</function></funcdef>
|
|
<paramdef>int <parameter>conn</parameter></paramdef>
|
|
</funcsynopsis>
|
|
<para>
|
|
<function>OCIPLogin</function> Creates a persistent connection to an Oracle 8 database
|
|
and logs on. If the optional third parameter is not specified, PHP uses the environment
|
|
variable ORACLE_SID to determine which database to connect to.
|
|
</para>
|
|
<simpara>
|
|
See also <function>OCILogon</function> and
|
|
<function>OCINLogon</function>.
|
|
</refsect1>
|
|
|
|
<refentry id="function.ocinlogon">
|
|
<refnamediv>
|
|
<refname>OCINLogon</refname>
|
|
<refpurpose>Connect to an Oracle database and log on using a new connection.
|
|
Returns a new session.</refpurpose>
|
|
</refnamediv>
|
|
<refsect1>
|
|
<title>Description</title>
|
|
<funcsynopsis>
|
|
<funcdef>int <function>OCINLogon</function></funcdef>
|
|
<paramdef>int <parameter>conn</parameter></paramdef>
|
|
</funcsynopsis>
|
|
<para>
|
|
<function>OCINLogin</function> Creates a new connection to an Oracle 8 database
|
|
and logs on. If the optional third parameter is not specified, PHP uses the environment
|
|
variable ORACLE_SID to determine which database to connect to. <function>OCINLogon</function>
|
|
forces a new connection. This is should be used if you need to isolate a set of transactions.
|
|
By default, connections are shared at the page level if using <function>OCILogon</function>
|
|
or at the web server process level if using <function>OCIPLogon</function>. If you have
|
|
multiple connections open using <function>OCINLogon</function>, all commits and
|
|
rollbacks apply to the specified connection only..
|
|
</para>
|
|
<para>
|
|
This example demonstrates how the connections are separated.
|
|
<example>
|
|
<title>OCINLogon</title>
|
|
<programlisting>
|
|
<?php
|
|
print "<HTML><PRE>";
|
|
$db = "";
|
|
|
|
$c1 = ocilogon("scott","tiger",$db);
|
|
$c2 = ocinlogon("scott","tiger",$db);
|
|
|
|
function create_table($conn)
|
|
{ $stmt = ociparse($conn,"create table scott.hallo (test
|
|
varchar2(32))");
|
|
ociexecute($stmt);
|
|
echo $conn." created table\n\n";
|
|
}
|
|
|
|
function drop_table($conn)
|
|
{ $stmt = ociparse($conn,"drop table scott.hallo");
|
|
ociexecute($stmt);
|
|
echo $conn." dropped table\n\n";
|
|
}
|
|
|
|
function insert_data($conn)
|
|
{ $stmt = ociparse($conn,"insert into scott.hallo values($conn || ' ' || to_char(sysdate,'DD-MON-YY HH24:MI:SS'))");
|
|
ociexecute($stmt,OCI_DEFAULT);
|
|
echo $conn." inserted hallo\n\n";
|
|
}
|
|
|
|
function delete_data($conn)
|
|
{ $stmt = ociparse($conn,"delete from scott.hallo");
|
|
ociexecute($stmt,OCI_DEFAULT);
|
|
echo $conn." deleted hallo\n\n";
|
|
}
|
|
|
|
function commit($conn)
|
|
{ ocicommit($conn);
|
|
echo $conn." commited\n\n";
|
|
}
|
|
|
|
function rollback($conn)
|
|
{ ocirollback($conn);
|
|
echo $conn." rollback\n\n";
|
|
}
|
|
|
|
function select_data($conn)
|
|
{ $stmt = ociparse($conn,"select * from scott.hallo");
|
|
ociexecute($stmt,OCI_DEFAULT);
|
|
echo $conn."----selecting\n\n";
|
|
while (ocifetch($stmt))
|
|
echo $conn." <".ociresult($stmt,"TEST").">\n\n";
|
|
echo $conn."----done\n\n";
|
|
}
|
|
|
|
create_table($c1);
|
|
insert_data($c1);
|
|
|
|
select_data($c1);
|
|
select_data($c2);
|
|
|
|
rollback($c1);
|
|
|
|
select_data($c1);
|
|
select_data($c2);
|
|
|
|
insert_data($c2);
|
|
commit($c2);
|
|
|
|
select_data($c1);
|
|
|
|
delete_data($c1);
|
|
select_data($c1);
|
|
select_data($c2);
|
|
commit($c1);
|
|
|
|
select_data($c1);
|
|
select_data($c2);
|
|
|
|
|
|
drop_table($c1);
|
|
print "</PRE></HTML>";
|
|
?></programlisting></example>
|
|
<simpara>
|
|
See also <function>OCILogon</function> and
|
|
<function>OCIPLogon</function>.
|
|
</refsect1>
|
|
|
|
<refentry id="function.ocilogoff">
|
|
<refnamediv>
|
|
<refname>OCILogOff</refname>
|
|
<refpurpose>Disconnects from Oracle</refpurpose>
|
|
</refnamediv>
|
|
<refsect1>
|
|
<title>Description</title>
|
|
<funcsynopsis>
|
|
<funcdef>int <function>OCILogOff</function></funcdef>
|
|
<paramdef>int <parameter>connection</parameter></paramdef>
|
|
</funcsynopsis>
|
|
<para>
|
|
<function>OCILogOff</function> closes an Oracle connection.
|
|
</para>
|
|
</refsect1>
|
|
</refentry>
|
|
|
|
<refentry id="function.ociexecute">
|
|
<refnamediv>
|
|
<refname>OCIExecute</refname>
|
|
<refpurpose>Execute a statement</refpurpose>
|
|
</refnamediv>
|
|
<refsect1>
|
|
<title>Description</title>
|
|
<funcsynopsis>
|
|
<funcdef>int <function>OCIExecute</function></funcdef>
|
|
<paramdef>int <parameter>statement</parameter></paramdef>
|
|
<paramdef>int <parameter><optional>mode</optional></parameter></paramdef>
|
|
</funcsynopsis>
|
|
<para>
|
|
<function>OCIExecute</function> executes a previously parsed statement.
|
|
(see <function>OCIParse</function>). The optional <parameter>mode</parameter>
|
|
allows you to specify the execution-mode (default is OCI_COMMIT_ON_SUCCESS).
|
|
If you don't want statements to be commited automaticly specify OCI_DEFAULT as
|
|
your mode.
|
|
</para>
|
|
</refsect1>
|
|
</refentry>
|
|
|
|
<refentry id="function.ocicommit">
|
|
<refnamediv>
|
|
<refname>OCICommit</refname>
|
|
<refpurpose>Commits outstanding transactions</refpurpose>
|
|
</refnamediv>
|
|
<refsect1>
|
|
<title>Description</title>
|
|
<funcsynopsis>
|
|
<funcdef>int <function>OCICommit</function></funcdef>
|
|
<paramdef>int <parameter>connection</parameter></paramdef>
|
|
</funcsynopsis>
|
|
<para>
|
|
<function>OCICommit</function> commits all outstanding statements for
|
|
Oracle connection <parameter>connection</parameter>.
|
|
</para>
|
|
</refsect1>
|
|
</refentry>
|
|
|
|
<refentry id="function.ocirollback">
|
|
<refnamediv>
|
|
<refname>OCIRollback</refname>
|
|
<refpurpose>Rolls back outstanding transactions</refpurpose>
|
|
</refnamediv>
|
|
<refsect1>
|
|
<title>Description</title>
|
|
<funcsynopsis>
|
|
<funcdef>int <function>OCIRollback</function></funcdef>
|
|
<paramdef>int <parameter>connection</parameter></paramdef>
|
|
</funcsynopsis>
|
|
<para>
|
|
<function>OCICommit</function> rolls back all outstanding statements for
|
|
Oracle connection <parameter>connection</parameter>.
|
|
</para>
|
|
</refsect1>
|
|
</refentry>
|
|
|
|
<refentry id="function.ocinewdescriptor">
|
|
<refnamediv>
|
|
<refname>OCINewDescriptor</refname>
|
|
<refpurpose>Initialize a new empty descriptor LOB/FILE (LOB is default)</refpurpose>
|
|
</refnamediv>
|
|
<refsect1>
|
|
<title>Description</title>
|
|
<funcsynopsis>
|
|
<funcdef>string <function>OCINewDescriptor</function></funcdef>
|
|
<paramdef>int <parameter>connection</parameter></paramdef>
|
|
<paramdef>int <parameter><optional>type</optional></parameter></paramdef>
|
|
</funcsynopsis>
|
|
<para>
|
|
<function>OCINewDescriptor</function> Allocates storage to hold descriptors or LOB locators.
|
|
Valid values for the valid <parameter>type</parameter> are OCI_D_FILE, OCI_D_LOB, OCI_D_ROWID.
|
|
|
|
</para>
|
|
<example>
|
|
<title>OCINewDescriptor</title>
|
|
<programlisting>
|
|
<?php
|
|
/* This script is designed to be called from a HTML form.
|
|
* It expects $user, $password, $table, $where, and $commitsize
|
|
* to be passed in from the form. The script then deletes
|
|
* the selected rows using the ROWID and commits after each
|
|
* set of $commitsize rows. (Use with care, there is no rollback)
|
|
*/
|
|
$conn = OCILogon($user, $password);
|
|
$stmt = OCIParse($conn,"select rowid from $table $where");
|
|
$rowid = OCINewDescriptor($conn,OCI_D_ROWID);
|
|
OCIDefineByName($stmt,"ROWID",&$rowid);
|
|
OCIExecute($stmt);
|
|
while ( OCIFetch($stmt) ) {
|
|
$nrows = OCIRowCount($stmt);
|
|
$delete = OCIParse($conn,"delete from $table where ROWID = :rid");
|
|
OCIBindByName($delete,":rid",&$rowid,-1,OCI_B_ROWID);
|
|
OCIExecute($delete);
|
|
print "$nrows\n";
|
|
if ( ($nrows % $commitsize) == 0 ) {
|
|
OCICommit($conn);
|
|
}
|
|
}
|
|
$nrows = OCIRowCount($stmt);
|
|
print "$nrows deleted...\n";
|
|
OCIFreeStatement($stmt);
|
|
OCILogoff($conn);
|
|
?>
|
|
</programlisting></example>
|
|
|
|
</refsect1>
|
|
</refentry>
|
|
<refentry id="function.ocirowcount">
|
|
<refnamediv>
|
|
<refname>OCIRowCount</refname>
|
|
<refpurpose>Gets the number of affected rows</refpurpose>
|
|
</refnamediv>
|
|
<refsect1>
|
|
<title>Description</title>
|
|
<funcsynopsis>
|
|
<funcdef>int <function>OCIRowCount</function></funcdef>
|
|
<paramdef>int <parameter>statement</parameter></paramdef>
|
|
</funcsynopsis>
|
|
<para>
|
|
<function>OCIRowCounts</function> returns the number of rows affected
|
|
for eg update-statements. This funtions will not tell you the number
|
|
of rows that a select will return!
|
|
<para>
|
|
<example>
|
|
<title>OCIRowCount</title>
|
|
<programlisting>
|
|
<?php
|
|
print "<HTML><PRE>";
|
|
$conn = OCILogon("scott","tiger");
|
|
$stmt = OCIParse($conn,"create table emp2 as select * from emp");
|
|
OCIExecute($stmt);
|
|
print OCIRowCount($stmt) . " rows inserted.<BR>";
|
|
OCIFreeStatement($stmt);
|
|
$stmt = OCIParse($conn,"delete from emp2");
|
|
OCIExecute($stmt);
|
|
print OCIRowCount($stmt) . " rows deleted.<BR>";
|
|
OCICommit($conn);
|
|
OCIFreeStatement($stmt);
|
|
$stmt = OCIParse($conn,"drop table emp2");
|
|
OCIExecute($stmt);
|
|
OCIFreeStatement($stmt);
|
|
OCILogOff($conn);
|
|
print "</PRE></HTML>";
|
|
?> </programlisting></example>
|
|
|
|
</refsect1>
|
|
</refentry>
|
|
|
|
<refentry id="function.ocinumcols">
|
|
<refnamediv>
|
|
<refname>OCINumCols</refname>
|
|
<refpurpose>Return the number of result columns in a statement</refpurpose>
|
|
</refnamediv>
|
|
<refsect1>
|
|
<title>Description</title>
|
|
<funcsynopsis>
|
|
<funcdef>int <function>OCINumCols</function></funcdef>
|
|
<paramdef>int <parameter>stmt</parameter></paramdef>
|
|
</funcsynopsis>
|
|
<para>
|
|
<function>OCINumCols</function> returns the number of columns in a statement</para>
|
|
<example>
|
|
<title>OCINumCols</title>
|
|
<programlisting>
|
|
<?php
|
|
print "<HTML><PRE>\n";
|
|
$conn = OCILogon("scott", "tiger");
|
|
$stmt = OCIParse($conn,"select * from emp");
|
|
OCIExecute($stmt);
|
|
while ( OCIFetch($stmt) ) {
|
|
print "\n";
|
|
$ncols = OCINumCols($stmt);
|
|
for ( $i = 1; $i <= $ncols; $i++ ) {
|
|
$column_name = OCIColumnName($stmt,$i);
|
|
$column_value = OCIResult($stmt,$i);
|
|
print $column_name . ': ' . $column_value . "\n";
|
|
}
|
|
print "\n";
|
|
}
|
|
OCIFreeStatement($stmt);
|
|
OCILogoff($conn);
|
|
print "</PRE>";
|
|
print "</HTML>\n";
|
|
?> </programlisting></example>
|
|
|
|
</refsect1>
|
|
</refentry>
|
|
|
|
<refentry id="function.ociresult">
|
|
<refnamediv>
|
|
<refname>OCIResult</refname>
|
|
<refpurpose>Returns coulumn value for fetched row</refpurpose>
|
|
</refnamediv>
|
|
<refsect1>
|
|
<title>Description</title>
|
|
<funcsynopsis>
|
|
<funcdef>int <function>OCIResult</function></funcdef>
|
|
<paramdef>int <parameter>statement</parameter></paramdef>
|
|
<paramdef>mixed <parameter>column</parameter></paramdef>
|
|
</funcsynopsis>
|
|
<para>
|
|
<function>OCIResult</function> returns the data for column
|
|
<parameter>column</parameter> in the current row (see
|
|
<function>OCIFetch</function>).<function>OCIResult</function> will
|
|
return everything as strings except for abstract types (ROWIDs, LOBs and FILEs).
|
|
</para>
|
|
</refsect1>
|
|
</refentry>
|
|
|
|
<refentry id="function.ocifetch">
|
|
<refnamediv>
|
|
<refname>OCIFetch</refname>
|
|
<refpurpose>Fetches the next row into result-buffer</refpurpose>
|
|
</refnamediv>
|
|
<refsect1>
|
|
<title>Description</title>
|
|
<funcsynopsis>
|
|
<funcdef>int <function>OCIFetch</function></funcdef>
|
|
<paramdef>int <parameter>statement</parameter></paramdef>
|
|
</funcsynopsis>
|
|
<para>
|
|
<function>OCIFetch</function> fetches the next row (for SELECT statements)
|
|
into the internal result-buffer.
|
|
</para>
|
|
</refsect1>
|
|
</refentry>
|
|
|
|
<refentry id="function.ocifetchinto">
|
|
<refnamediv>
|
|
<refname>OCIFetchInto</refname>
|
|
<refpurpose>Fetches the next row into result-array</refpurpose>
|
|
</refnamediv>
|
|
<refsect1>
|
|
<title>Description</title>
|
|
<funcsynopsis>
|
|
<funcdef>int <function>OCIFetchInto</function></funcdef>
|
|
<paramdef>int <parameter>stmt</parameter></paramdef>
|
|
<paramdef>array &<parameter>result</parameter></paramdef>
|
|
<paramdef>int <parameter><optional>mode</optional></parameter></paramdef>
|
|
</funcsynopsis>
|
|
<para>
|
|
<function>OCIFetchInto</function> fetches the next row (for SELECT statements)
|
|
into the <parameter>result</parameter> array. <function>OCIFetchInto</function>
|
|
will overwrite the previous content of <parameter>result</parameter>. By default
|
|
<parameter>result</parameter> will contain a one-based array of all columns
|
|
that are not NULL.
|
|
</para>
|
|
<para>
|
|
The <parameter>mode</parameter> parameter allows you to change the default
|
|
behaviour. You can specify more than one flag by simply addig them up
|
|
(eg OCI_ASSOC+OCI_RETURN_NULLS). The known flags are:
|
|
|
|
<simplelist>
|
|
<member><literal>OCI_ASSOC</literal> Return an associative array.</member>
|
|
<member><literal>OCI_NUM</literal> Return an numbered array
|
|
starting with one. (DEFAULT)</member>
|
|
<member><literal>OCI_RETURN_NULLS</literal> Return empty columns.</member>
|
|
<member><literal>OCI_RETURN_LOBS</literal> Return the value of a LOB instead of the desxriptor.</member>
|
|
</simplelist>
|
|
|
|
</para>
|
|
<para>
|
|
</para>
|
|
</refsect1>
|
|
</refentry>
|
|
|
|
<refentry id="function.ocifetchstatement">
|
|
<refnamediv>
|
|
<refname>OCIFetchStatement</refname>
|
|
<refpurpose>Fetch all rows of result data into an array.</refpurpose>
|
|
</refnamediv>
|
|
<refsect1>
|
|
<title>Description</title>
|
|
<funcsynopsis>
|
|
<funcdef>int <function>OCIFetchStatement</function></funcdef>
|
|
<paramdef>int <parameter>stmt</parameter></paramdef>
|
|
<paramdef>array &<parameter>variable</parameter></paramdef>
|
|
</funcsynopsis>
|
|
<para>
|
|
<function>OCIFetchStatement</function> fetches all the rows from a
|
|
result into a user-defined array. <function>OCIFetchStatement</function>
|
|
returns the number of rows fetched.
|
|
</para>
|
|
<example>
|
|
<title>OCIFetchStatement</title>
|
|
<programlisting>
|
|
<?php
|
|
/* OCIFetchStatement example mbritton@verinet.com (990624) */
|
|
|
|
$conn = OCILogon("scott","tiger");
|
|
|
|
$stmt = OCIParse($conn,"select * from emp");
|
|
|
|
OCIExecute($stmt);
|
|
|
|
$nrows = OCIFetchStatement($stmt,$results);
|
|
if ( $nrows > 0 ) {
|
|
print "<TABLE BORDER=\"1\">\n";
|
|
print "<TR>\n";
|
|
while ( list( $key, $val ) = each( $results ) ) {
|
|
print "<TH>$key</TH>\n";
|
|
}
|
|
print "</TR>\n";
|
|
|
|
for ( $i = 0; $i < $nrows; $i++ ) {
|
|
reset($results);
|
|
print "<TR>\n";
|
|
while ( $column = each($results) ) {
|
|
$data = $column['value'];
|
|
print "<TD>$data[$i]</TD>\n";
|
|
}
|
|
print "</TR>\n";
|
|
}
|
|
print "</TABLE>\n";
|
|
} else {
|
|
echo "No data found<BR>\n";
|
|
}
|
|
print "$nrows Records Selected<BR>\n";
|
|
|
|
OCIFreeStatement($stmt);
|
|
OCILogoff($conn);
|
|
|
|
?></programlisting></example>
|
|
|
|
</refsect1>
|
|
</refentry>
|
|
<refentry id="function.ocicolumnisnull">
|
|
<refnamediv>
|
|
<refname>OCIColumnIsNULL</refname>
|
|
<refpurpose>test whether a result column is NULL</refpurpose>
|
|
</refnamediv>
|
|
<refsect1>
|
|
<title>Description</title>
|
|
<funcsynopsis>
|
|
<funcdef>int <function>OCIColumnIsNULL</function></funcdef>
|
|
<paramdef>int <parameter>stmt</parameter></paramdef>
|
|
<paramdef>mixed <parameter>column</parameter></paramdef>
|
|
</funcsynopsis>
|
|
<para>
|
|
<function>OCIColumnIsNULL</function> returns true if the returned
|
|
column <parameter>col</parameter> in the result from the
|
|
statement <parameter>stmt</parameter> is NULL. You can either use
|
|
the column-number (1-Based) or the column-name for the <parameter>col</parameter>
|
|
parameter.
|
|
</para>
|
|
</refsect1>
|
|
</refentry>
|
|
|
|
|
|
<refentry id="function.ocicolumnsize">
|
|
<refnamediv>
|
|
<refname>OCIColumnSize</refname>
|
|
<refpurpose>return result column size</refpurpose>
|
|
</refnamediv>
|
|
<refsect1>
|
|
<title>Description</title>
|
|
<funcsynopsis>
|
|
<funcdef>int <function>OCIColumnSize</function></funcdef>
|
|
<paramdef>int <parameter>stmt</parameter></paramdef>
|
|
<paramdef>mixed <parameter>column</parameter></paramdef>
|
|
</funcsynopsis>
|
|
<para>
|
|
<function>OCIColumnSize</function> returns the size of the column
|
|
as given by Oracle. You can either use
|
|
the column-number (1-Based) or the column-name for the <parameter>col</parameter>
|
|
parameter.
|
|
</para>
|
|
<para>
|
|
<example>
|
|
<title>OCIColumnSize</title>
|
|
<programlisting>
|
|
<?php
|
|
print "<HTML><PRE>\n";
|
|
$conn = OCILogon("scott", "tiger");
|
|
$stmt = OCIParse($conn,"select * from emp");
|
|
OCIExecute($stmt);
|
|
print "<TABLE BORDER=\"1\">";
|
|
print "<TR>";
|
|
print "<TH>Name</TH>";
|
|
print "<TH>Type</TH>";
|
|
print "<TH>Length</TH>";
|
|
print "</TR>";
|
|
$ncols = OCINumCols($stmt);
|
|
for ( $i = 1; $i <= $ncols; $i++ ) {
|
|
$column_name = OCIColumnName($stmt,$i);
|
|
$column_type = OCIColumnType($stmt,$i);
|
|
$column_size = OCIColumnSize($stmt,$i);
|
|
print "<TR>";
|
|
print "<TD>$column_name</TD>";
|
|
print "<TD>$column_type</TD>";
|
|
print "<TD>$column_size</TD>";
|
|
print "</TR>";
|
|
}
|
|
OCIFreeStatement($stmt);
|
|
OCILogoff($conn);
|
|
print "</PRE>";
|
|
print "</HTML>\n";
|
|
?> </programlisting></example>
|
|
<simpara>
|
|
See also <function>OCINumCols</function>, <function>OCIColumnName</function>,
|
|
and <function>OCIColumnSize</function>.
|
|
</refsect1>
|
|
</refentry>
|
|
|
|
<refentry id="function.ociserverversion">
|
|
<refnamediv>
|
|
<refname>OCIServerVersion</refname>
|
|
<refpurpose>Return a string containing server version information.</refpurpose>
|
|
</refnamediv>
|
|
<refsect1>
|
|
<title>Description</title>
|
|
<funcsynopsis>
|
|
<funcdef>string <function>OCIServerVersion</function></funcdef>
|
|
<paramdef>int <parameter>conn</parameter></paramdef>
|
|
</funcsynopsis>
|
|
<para>
|
|
<example>
|
|
<title>OCIServerVersion</title>
|
|
<programlisting>
|
|
<?php
|
|
$conn = OCILogon("scott","tiger");
|
|
print "Server Version: " . OCIServerVersion($conn);
|
|
OCILogOff($conn);
|
|
?></programlisting></example>
|
|
|
|
</refsect1>
|
|
</refentry>
|
|
|
|
<refentry id="function.ocistatementtype">
|
|
<refnamediv>
|
|
<refname>OCIStatementType</refname>
|
|
<refpurpose>Return the type of an OCI statement.</refpurpose>
|
|
</refnamediv>
|
|
<refsect1>
|
|
<title>Description</title>
|
|
<funcsynopsis>
|
|
<funcdef>string <function>OCIStatementType</function></funcdef>
|
|
<paramdef>int <parameter>stmt</parameter></paramdef>
|
|
</funcsynopsis>
|
|
<para>
|
|
<function>OCIStatementType</function> returns on of the following values:
|
|
<orderedlist>
|
|
<listitem><simpara> "SELECT"
|
|
<listitem><simpara> "UPDATE"
|
|
<listitem><simpara> "DELETE"
|
|
<listitem><simpara> "INSERT"
|
|
<listitem><simpara> "CREATE"
|
|
<listitem><simpara> "DROP"
|
|
<listitem><simpara> "ALTER"
|
|
<listitem><simpara> "BEGIN"
|
|
<listitem><simpara> "DECLARE"
|
|
<listitem><simpara> "UNKNOWN"
|
|
</orderedlist>
|
|
<para>
|
|
<example>
|
|
<title>Code examples</title>
|
|
<programlisting>
|
|
<?php
|
|
print "<HTML><PRE>";
|
|
$conn = OCILogon("scott","tiger");
|
|
$sql = "delete from emp where deptno = 10";
|
|
|
|
$stmt = OCIParse($conn,$sql);
|
|
if ( OCIStatementType($stmt) == "DELETE" ) {
|
|
die "You are not allowed to delete from this table<BR>";
|
|
}
|
|
|
|
OCILogoff($conn);
|
|
print "</PRE></HTML>";
|
|
?>
|
|
</programlisting></example>
|
|
|
|
</refsect1>
|
|
</refentry>
|
|
|
|
<refentry id="function.ocinewcursor">
|
|
<refnamediv>
|
|
<refname>OCINewCursor</refname>
|
|
<refpurpose>return a new cursor (Statement-Handle) - use this to bind ref-cursors!</refpurpose>
|
|
</refnamediv>
|
|
<refsect1>
|
|
<title>Description</title>
|
|
<funcsynopsis>
|
|
<funcdef>int <function>OCINewCursor</function></funcdef>
|
|
<paramdef>int <parameter>conn</parameter></paramdef>
|
|
</funcsynopsis>
|
|
<para>
|
|
<function>OCINewCursor</function> allocates a new statement handle on the specified
|
|
connection.
|
|
</para>
|
|
<para>
|
|
<example>
|
|
<title>Using a REF CURSOR from a stored procedure</title>
|
|
<programlisting>
|
|
<?php
|
|
// suppose your stored procedure info.output returns a ref cursor in :data
|
|
|
|
$conn = OCILogon("scott","tiger");
|
|
$curs = OCINewCursor($conn);
|
|
$stmt = OCIParse($conn,"begin info.output(:data); end;");
|
|
|
|
ocibindbyname($stmt,"data",&$curs,-1,OCI_B_CURSOR);
|
|
ociexecute($stmt);
|
|
ociexecute($curs);
|
|
|
|
while (OCIFetchInto($curs,&$data)) {
|
|
var_dump($data);
|
|
}
|
|
|
|
OCIFreeCursor($stmt);
|
|
OCIFreeStatement($curs);
|
|
OCILogoff($conn);
|
|
?></programlisting></example>
|
|
|
|
<para>
|
|
<example>
|
|
<title>Using a REF CURSOR in a select statement</title>
|
|
<programlisting>
|
|
<?php
|
|
print "<HTML><BODY>";
|
|
$conn = OCILogon("scott","tiger");
|
|
$count_cursor = "CURSOR(select count(empno) num_emps from emp " .
|
|
"where emp.deptno = dept.deptno) as EMPCNT from dept";
|
|
$stmt = OCIParse($conn,"select deptno,dname,$count_cursor");
|
|
|
|
ociexecute($stmt);
|
|
print "<TABLE BORDER=\"1\">";
|
|
print "<TR>";
|
|
print "<TH>DEPT NAME</TH>";
|
|
print "<TH>DEPT #</TH>";
|
|
print "<TH># EMPLOYEES</TH>";
|
|
print "</TR>";
|
|
|
|
while (OCIFetchInto($stmt,&$data,OCI_ASSOC)) {
|
|
print "<TR>";
|
|
$dname = $data["DNAME"];
|
|
$deptno = $data["DEPTNO"];
|
|
print "<TD>$dname</TD>";
|
|
print "<TD>$deptno</TD>";
|
|
ociexecute($data[ "EMPCNT" ]);
|
|
while (OCIFetchInto($data[ "EMPCNT" ],&$subdata,OCI_ASSOC)) {
|
|
$num_emps = $subdata["NUM_EMPS"];
|
|
print "<TD>$num_emps</TD>";
|
|
}
|
|
print "</TR>";
|
|
}
|
|
print "</TABLE>";
|
|
print "</BODY></HTML>";
|
|
OCIFreeStatement($stmt);
|
|
OCILogoff($conn);
|
|
?></programlisting></example>
|
|
|
|
</refsect1>
|
|
</refentry>
|
|
|
|
<refentry id="function.ocifreestatement">
|
|
<refnamediv>
|
|
<refname>OCIFreeStatement</refname>
|
|
<refpurpose>Free all resources associated with a statement.</refpurpose>
|
|
</refnamediv>
|
|
<refsect1>
|
|
<title>Description</title>
|
|
<funcsynopsis>
|
|
<funcdef>int <function>OCIFreeStatement</function></funcdef>
|
|
<paramdef>int <parameter>stmt</parameter></paramdef>
|
|
</funcsynopsis>
|
|
<para>
|
|
<function>OCIFreeStatement</function> returns true if successful, or false if
|
|
unsuccessful.
|
|
</para>
|
|
</refsect1>
|
|
</refentry>
|
|
|
|
<refentry id="function.ocifreecursor">
|
|
<refnamediv>
|
|
<refname>OCIFreeCursor</refname>
|
|
<refpurpose>Free all resources associated with a cursor.</refpurpose>
|
|
</refnamediv>
|
|
<refsect1>
|
|
<title>Description</title>
|
|
<funcsynopsis>
|
|
<funcdef>int <function>OCIFreeCursor</function></funcdef>
|
|
<paramdef>int <parameter>stmt</parameter></paramdef>
|
|
</funcsynopsis>
|
|
<para>
|
|
<function>OCIFreeCursor</function> returns true if successful, or false if
|
|
unsuccessful.
|
|
</para>
|
|
</refsect1>
|
|
</refentry>
|
|
|
|
<refentry id="function.ocicolumnname">
|
|
<refnamediv>
|
|
<refname>OCIColumnName</refname>
|
|
<refpurpose>Returns the name of a column.</refpurpose>
|
|
</refnamediv>
|
|
<refsect1>
|
|
<title>Description</title>
|
|
<funcsynopsis>
|
|
<funcdef>string <function>OCIColumnName</function></funcdef>
|
|
<paramdef>int <parameter>stmt</parameter></paramdef>
|
|
<paramdef>int <parameter>col</parameter></paramdef>
|
|
</funcsynopsis>
|
|
<simpara>
|
|
<function>OCIColumnName</function> returns the name of the column
|
|
corresponding to the column number (1-based) that is passed in.
|
|
|
|
<para>
|
|
<example>
|
|
<title>OCIColumnName</title>
|
|
<programlisting>
|
|
<?php
|
|
print "<HTML><PRE>\n";
|
|
$conn = OCILogon("scott", "tiger");
|
|
$stmt = OCIParse($conn,"select * from emp");
|
|
OCIExecute($stmt);
|
|
print "<TABLE BORDER=\"1\">";
|
|
print "<TR>";
|
|
print "<TH>Name</TH>";
|
|
print "<TH>Type</TH>";
|
|
print "<TH>Length</TH>";
|
|
print "</TR>";
|
|
$ncols = OCINumCols($stmt);
|
|
for ( $i = 1; $i <= $ncols; $i++ ) {
|
|
$column_name = OCIColumnName($stmt,$i);
|
|
$column_type = OCIColumnType($stmt,$i);
|
|
$column_size = OCIColumnSize($stmt,$i);
|
|
print "<TR>";
|
|
print "<TD>$column_name</TD>";
|
|
print "<TD>$column_type</TD>";
|
|
print "<TD>$column_size</TD>";
|
|
print "</TR>";
|
|
}
|
|
OCIFreeStatement($stmt);
|
|
OCILogoff($conn);
|
|
print "</PRE>";
|
|
print "</HTML>\n";
|
|
?> </programlisting></example>
|
|
<simpara>
|
|
See also <function>OCINumCols</function>, <function>OCIColumnType</function>,
|
|
and <function>OCIColumnSize</function>.
|
|
</refsect1>
|
|
</refentry>
|
|
|
|
<refentry id="function.ocicolumntype">
|
|
<refnamediv>
|
|
<refname>OCIColumnType</refname>
|
|
<refpurpose>Returns the data type of a column.</refpurpose>
|
|
</refnamediv>
|
|
<refsect1>
|
|
<title>Description</title>
|
|
<funcsynopsis>
|
|
<funcdef>mixed <function>OCIColumnName</function></funcdef>
|
|
<paramdef>int <parameter>stmt</parameter></paramdef>
|
|
<paramdef>int <parameter>col</parameter></paramdef>
|
|
</funcsynopsis>
|
|
<simpara>
|
|
<function>OCIColumnType</function> returns the data type of the column
|
|
corresponding to the column number (1-based) that is passed in.
|
|
|
|
<para>
|
|
<example>
|
|
<title>OCIColumnType</title>
|
|
<programlisting>
|
|
<?php
|
|
print "<HTML><PRE>\n";
|
|
$conn = OCILogon("scott", "tiger");
|
|
$stmt = OCIParse($conn,"select * from emp");
|
|
OCIExecute($stmt);
|
|
print "<TABLE BORDER=\"1\">";
|
|
print "<TR>";
|
|
print "<TH>Name</TH>";
|
|
print "<TH>Type</TH>";
|
|
print "<TH>Length</TH>";
|
|
print "</TR>";
|
|
$ncols = OCINumCols($stmt);
|
|
for ( $i = 1; $i <= $ncols; $i++ ) {
|
|
$column_name = OCIColumnName($stmt,$i);
|
|
$column_type = OCIColumnType($stmt,$i);
|
|
$column_size = OCIColumnSize($stmt,$i);
|
|
print "<TR>";
|
|
print "<TD>$column_name</TD>";
|
|
print "<TD>$column_type</TD>";
|
|
print "<TD>$column_size</TD>";
|
|
print "</TR>";
|
|
}
|
|
OCIFreeStatement($stmt);
|
|
OCILogoff($conn);
|
|
print "</PRE>";
|
|
print "</HTML>\n";
|
|
?> </programlisting></example>
|
|
<simpara>
|
|
See also <function>OCINumCols</function>, <function>OCIColumnName</function>,
|
|
and <function>OCIColumnSize</function>.
|
|
|
|
</refsect1>
|
|
</refentry>
|
|
<refentry id="function.ociparse">
|
|
<refnamediv>
|
|
<refname>OCIParse</refname>
|
|
<refpurpose>Parse a query and return a statement</refpurpose>
|
|
</refnamediv>
|
|
<refsect1>
|
|
<title>Description</title>
|
|
<funcsynopsis>
|
|
<funcdef>int <function>OCIParse</function></funcdef>
|
|
<paramdef>int <parameter>conn</parameter></paramdef>
|
|
<paramdef>strint <parameter>query</parameter></paramdef>
|
|
</funcsynopsis>
|
|
<simpara>
|
|
<function>OCIParse</function> parses the <parameter>query</parameter>
|
|
using <parameter>conn</parameter>. It returns true if the query is
|
|
valid, false if not. The <parameter>query</parameter> can be any valid
|
|
SQL statement.
|
|
</refsect1>
|
|
</refentry>
|
|
<refentry id="function.ocierror">
|
|
<refnamediv>
|
|
<refname>OCIError</refname>
|
|
<refpurpose>Return the last error of stmt|conn|global.
|
|
If no error happened returns false.
|
|
</refpurpose>
|
|
</refnamediv>
|
|
<refsect1>
|
|
<title>Description</title>
|
|
<funcsynopsis>
|
|
<funcdef>int <function>OCIError</function></funcdef>
|
|
<paramdef>int <parameter><optional>stmt|conn</optional></parameter></paramdef>
|
|
</funcsynopsis>
|
|
<simpara>
|
|
<function>OCIError</function> returns the last error found. If the optional
|
|
<parameter>stmt|conn</parameter> is not provided, the last error encountered
|
|
is returned. If no error is found, <function>OCIError</function> returns false.
|
|
</refsect1>
|
|
</refentry>
|
|
<refentry id="function.ociinternaldebug">
|
|
<refnamediv>
|
|
<refname>OCIInternalDebug</refname>
|
|
<refpurpose>Enables or disables internal debug output. By default it is disabled</refpurpose>
|
|
</refnamediv>
|
|
<refsect1>
|
|
<title>Description</title>
|
|
<funcsynopsis>
|
|
<funcdef>void <function>OCIInternalDebug</function></funcdef>
|
|
<paramdef>int <parameter>onoff</parameter></paramdef>
|
|
</funcsynopsis>
|
|
<simpara>
|
|
<function>OCIInternalDebug</function> enables internal debug output. Set <parameter>onoff</parameter>
|
|
to 0 to turn debug output off, 1 to turn it on.
|
|
</refsect1>
|
|
</refentry>
|
|
</reference>
|
|
|
|
<!-- Keep this comment at the end of the file
|
|
Local variables:
|
|
mode: sgml
|
|
sgml-omittag:t
|
|
sgml-shorttag:t
|
|
sgml-minimize-attributes:nil
|
|
sgml-always-quote-attributes:t
|
|
sgml-indent-step:1
|
|
sgml-indent-data:t
|
|
sgml-parent-document:nil
|
|
sgml-default-dtd-file:"../manual.ced"
|
|
sgml-exposed-tags:nil
|
|
sgml-local-catalogs:nil
|
|
sgml-local-ecat-files:nil
|
|
End:
|
|
-->
|