mirror of
https://github.com/sigmasternchen/php-doc-en
synced 2025-03-27 14:28:56 +00:00
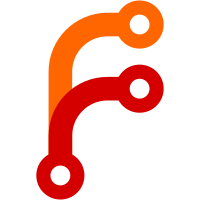
as UNIX is a registered trademark by the Open Group. References to UNIX were left in where it made sense, like example outputs, and so forth. You can read the Trademark User Guide for "UNIX" at: http://www.unix.org/tmug2.ps or http://www.unix.org/tmug2.pdf git-svn-id: https://svn.php.net/repository/phpdoc/en/trunk@147067 c90b9560-bf6c-de11-be94-00142212c4b1
256 lines
7.6 KiB
XML
256 lines
7.6 KiB
XML
<?xml version="1.0" encoding="iso-8859-1"?>
|
|
<!-- $Revision: 1.17 $ -->
|
|
<reference id="ref.sockets">
|
|
<title>Socket functions</title>
|
|
<titleabbrev>Sockets</titleabbrev>
|
|
|
|
<partintro>
|
|
|
|
<section id="sockets.intro">
|
|
&reftitle.intro;
|
|
<para>
|
|
The socket extension implements a low-level interface to the socket
|
|
communication functions based on the popular BSD sockets, providing the
|
|
possibility to act as a socket server as well as a client.
|
|
</para>
|
|
<para>
|
|
For a more generic client-side socket interface, see
|
|
<function>stream_socket_client</function>,
|
|
<function>stream_socket_server</function>,
|
|
<function>fsockopen</function>, and
|
|
<function>pfsockopen</function>.
|
|
</para>
|
|
<para>
|
|
When using these functions, it is important to remember that while
|
|
many of them have identical names to their C counterparts, they
|
|
often have different declarations. Please be sure to read the
|
|
descriptions to avoid confusion.
|
|
</para>
|
|
<para>
|
|
Those unfamiliar with socket programming can find a lot
|
|
of useful material in the appropriate Unix man pages, and there is a great
|
|
deal of tutorial information on socket programming in C on the web, much
|
|
of which can be applied, with slight modifications, to socket programming
|
|
in PHP. The <ulink url="&url.socket.faq;">Unix Socket
|
|
FAQ</ulink> might be a good start.
|
|
</para>
|
|
&warn.experimental;
|
|
</section>
|
|
|
|
<section id="sockets.requirements">
|
|
&reftitle.required;
|
|
&no.requirement;
|
|
</section>
|
|
|
|
&reference.sockets.configure;
|
|
|
|
<section id="sockets.configuration">
|
|
&reftitle.runtime;
|
|
&no.config;
|
|
</section>
|
|
|
|
<section id="sockets.resources">
|
|
&reftitle.resources;
|
|
&no.resource;
|
|
</section>
|
|
|
|
&reference.sockets.constants;
|
|
|
|
<section id="sockets.errors">
|
|
<title>Socket Errors</title>
|
|
<para>
|
|
The socket extension was written to provide a useable interface to the
|
|
powerful BSD sockets. Care has been taken that the functions work equally
|
|
well on Win32 and Unix implementations. Almost all of the sockets
|
|
functions may fail under certain conditions and therefore emit an
|
|
<constant>E_WARNING</constant> message describing the error. Sometimes this
|
|
doesn't happen to the desire of the developer. For example the function
|
|
<function>socket_read</function> may suddenly emit an
|
|
<constant>E_WARNING</constant> message because the connection broke
|
|
unexpectedly. It's common to suppress the warning with the
|
|
<literal>@</literal>-operator and catch the error code within the
|
|
application with the <function>socket_last_error</function> function. You
|
|
may call the <function>socket_strerror</function> function with this error
|
|
code to retrieve a string describing the error. See their description for
|
|
more information.
|
|
</para>
|
|
<note>
|
|
<para>
|
|
The <constant>E_WARNING</constant> messages generated by the socket
|
|
extension are in English though the retrieved error message will appear
|
|
depending on the current locale (<constant>LC_MESSAGES</constant>):
|
|
<programlisting role="php">
|
|
<![CDATA[
|
|
Warning - socket_bind() unable to bind address [98]: Die Adresse wird bereits verwendet
|
|
]]>
|
|
</programlisting>
|
|
</para>
|
|
</note>
|
|
</section>
|
|
|
|
<section id="sockets.examples">
|
|
&reftitle.examples;
|
|
<para>
|
|
<example>
|
|
<title>Socket example: Simple TCP/IP server</title>
|
|
<para>
|
|
This example shows a simple talkback server. Change the
|
|
<varname>address</varname> and <varname>port</varname> variables
|
|
to suit your setup and execute. You may then connect to the
|
|
server with a command similar to: <command>telnet 192.168.1.53
|
|
10000</command> (where the address and port match your
|
|
setup). Anything you type will then be output on the server
|
|
side, and echoed back to you. To disconnect, enter 'quit'.
|
|
</para>
|
|
<programlisting role="php">
|
|
<![CDATA[
|
|
#!/usr/local/bin/php -q
|
|
<?php
|
|
error_reporting(E_ALL);
|
|
|
|
/* Allow the script to hang around waiting for connections. */
|
|
set_time_limit(0);
|
|
|
|
/* Turn on implicit output flushing so we see what we're getting
|
|
* as it comes in. */
|
|
ob_implicit_flush();
|
|
|
|
$address = '192.168.1.53';
|
|
$port = 10000;
|
|
|
|
if (($sock = socket_create(AF_INET, SOCK_STREAM, SOL_TCP)) < 0) {
|
|
echo "socket_create() failed: reason: " . socket_strerror($sock) . "\n";
|
|
}
|
|
|
|
if (($ret = socket_bind($sock, $address, $port)) < 0) {
|
|
echo "socket_bind() failed: reason: " . socket_strerror($ret) . "\n";
|
|
}
|
|
|
|
if (($ret = socket_listen($sock, 5)) < 0) {
|
|
echo "socket_listen() failed: reason: " . socket_strerror($ret) . "\n";
|
|
}
|
|
|
|
do {
|
|
if (($msgsock = socket_accept($sock)) < 0) {
|
|
echo "socket_accept() failed: reason: " . socket_strerror($msgsock) . "\n";
|
|
break;
|
|
}
|
|
/* Send instructions. */
|
|
$msg = "\nWelcome to the PHP Test Server. \n" .
|
|
"To quit, type 'quit'. To shut down the server type 'shutdown'.\n";
|
|
socket_write($msgsock, $msg, strlen($msg));
|
|
|
|
do {
|
|
if (false === ($buf = socket_read($msgsock, 2048, PHP_NORMAL_READ))) {
|
|
echo "socket_read() failed: reason: " . socket_strerror($ret) . "\n";
|
|
break 2;
|
|
}
|
|
if (!$buf = trim($buf)) {
|
|
continue;
|
|
}
|
|
if ($buf == 'quit') {
|
|
break;
|
|
}
|
|
if ($buf == 'shutdown') {
|
|
socket_close($msgsock);
|
|
break 2;
|
|
}
|
|
$talkback = "PHP: You said '$buf'.\n";
|
|
socket_write($msgsock, $talkback, strlen($talkback));
|
|
echo "$buf\n";
|
|
} while (true);
|
|
socket_close($msgsock);
|
|
} while (true);
|
|
|
|
socket_close($sock);
|
|
?>
|
|
]]>
|
|
</programlisting>
|
|
</example>
|
|
</para>
|
|
<para>
|
|
<example>
|
|
<title>Socket example: Simple TCP/IP client</title>
|
|
<para>
|
|
This example shows a simple, one-shot HTTP client. It simply
|
|
connects to a page, submits a HEAD request, echoes the reply,
|
|
and exits.
|
|
</para>
|
|
<programlisting role="php">
|
|
<![CDATA[
|
|
<?php
|
|
error_reporting(E_ALL);
|
|
|
|
echo "<h2>TCP/IP Connection</h2>\n";
|
|
|
|
/* Get the port for the WWW service. */
|
|
$service_port = getservbyname('www', 'tcp');
|
|
|
|
/* Get the IP address for the target host. */
|
|
$address = gethostbyname('www.example.com');
|
|
|
|
/* Create a TCP/IP socket. */
|
|
$socket = socket_create(AF_INET, SOCK_STREAM, SOL_TCP);
|
|
if ($socket < 0) {
|
|
echo "socket_create() failed: reason: " . socket_strerror($socket) . "\n";
|
|
} else {
|
|
echo "OK.\n";
|
|
}
|
|
|
|
echo "Attempting to connect to '$address' on port '$service_port'...";
|
|
$result = socket_connect($socket, $address, $service_port);
|
|
if ($result < 0) {
|
|
echo "socket_connect() failed.\nReason: ($result) " . socket_strerror($result) . "\n";
|
|
} else {
|
|
echo "OK.\n";
|
|
}
|
|
|
|
$in = "HEAD / HTTP/1.1\r\n";
|
|
$in .= "Host: www.example.com\r\n";
|
|
$in .= "Connection: Close\r\n\r\n";
|
|
$out = '';
|
|
|
|
echo "Sending HTTP HEAD request...";
|
|
socket_write($socket, $in, strlen($in));
|
|
echo "OK.\n";
|
|
|
|
echo "Reading response:\n\n";
|
|
while ($out = socket_read($socket, 2048)) {
|
|
echo $out;
|
|
}
|
|
|
|
echo "Closing socket...";
|
|
socket_close($socket);
|
|
echo "OK.\n\n";
|
|
?>
|
|
]]>
|
|
</programlisting>
|
|
</example>
|
|
</para>
|
|
</section>
|
|
</partintro>
|
|
|
|
&reference.sockets.functions;
|
|
|
|
</reference>
|
|
<!-- Keep this comment at the end of the file
|
|
Local variables:
|
|
mode: sgml
|
|
sgml-omittag:t
|
|
sgml-shorttag:t
|
|
sgml-minimize-attributes:nil
|
|
sgml-always-quote-attributes:t
|
|
sgml-indent-step:1
|
|
sgml-indent-data:t
|
|
indent-tabs-mode:nil
|
|
sgml-parent-document:nil
|
|
sgml-default-dtd-file:"../../../manual.ced"
|
|
sgml-exposed-tags:nil
|
|
sgml-local-catalogs:nil
|
|
sgml-local-ecat-files:nil
|
|
End:
|
|
vim600: syn=xml fen fdm=syntax fdl=2 si
|
|
vim: et tw=78 syn=sgml
|
|
vi: ts=1 sw=1
|
|
-->
|
|
|