mirror of
https://github.com/sigmasternchen/php-doc-en
synced 2025-03-16 00:48:54 +00:00
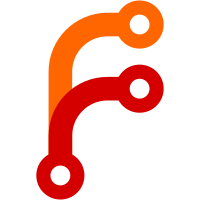
git-svn-id: https://svn.php.net/repository/phpdoc/en/trunk@64742 c90b9560-bf6c-de11-be94-00142212c4b1
287 lines
9.3 KiB
XML
Executable file
287 lines
9.3 KiB
XML
Executable file
<?xml version="1.0" encoding="iso-8859-1"?>
|
|
<!-- $Revision: 1.12 $ -->
|
|
<chapter id="faq.using">
|
|
<title>Using PHP</title>
|
|
<titleabbrev>Using PHP</titleabbrev>
|
|
|
|
<para>
|
|
This section gathers most common errors you can
|
|
face, while writing PHP scripts.
|
|
</para>
|
|
|
|
<qandaset>
|
|
<qandaentry id="faq.using.anyform">
|
|
<question>
|
|
<para>
|
|
I would like to write a generic PHP script that can handle data coming
|
|
from any form. How do I know which POST method variables are available?
|
|
</para>
|
|
</question>
|
|
<answer>
|
|
<para>
|
|
Make sure that the <link linkend="ini.track-vars">track_vars</link>
|
|
feature is enabled in your <filename>php.ini</filename>
|
|
file. Since PHP 4.0.3, this feature is always on. When
|
|
<literal>track_vars</literal> is on, it creates some
|
|
associative arrays, the most important here is:
|
|
<literal>$HTTP_POST_VARS</literal>. So, to write a generic
|
|
script to handle POST method variables you would
|
|
need something similar to the following:
|
|
<programlisting role="php">
|
|
<![CDATA[
|
|
foreach ($HTTP_POST_VARS as $var => $value) {
|
|
echo "$var = $value<br>\n";
|
|
}
|
|
]]>
|
|
</programlisting>
|
|
</para>
|
|
</answer>
|
|
</qandaentry>
|
|
|
|
<qandaentry id="faq.using.singlequotes">
|
|
<question>
|
|
<para>
|
|
I need to convert all single-quotes (') to a backslash
|
|
followed by a single-quote. How can I do this with a
|
|
regular expression?
|
|
</para>
|
|
</question>
|
|
<answer>
|
|
<para>
|
|
First off, take a look at the <function>addslashes</function>
|
|
function. It will do exactly what you want. You should also have
|
|
a look at the <link linkend="ini.magic-quotes-gpc">magic_quotes_gpc</link>
|
|
directive in your <filename>php.ini</filename> file.
|
|
</para>
|
|
</answer>
|
|
</qandaentry>
|
|
|
|
<qandaentry id="faq.using.wrong-order">
|
|
<question>
|
|
<para>
|
|
When I do the following, the output is printed in
|
|
the wrong order:
|
|
<programlisting role="php">
|
|
function myfunc($argument)
|
|
{
|
|
echo $argument + 10;
|
|
}
|
|
$variable = 10;
|
|
echo "myfunc($variable) = " . myfunc($variable);
|
|
</programlisting>
|
|
what's going on?
|
|
</para>
|
|
</question>
|
|
<answer>
|
|
<para>
|
|
To be able to use the results of your function in an expression (such
|
|
as concatenating it with other strings in the example above), you need
|
|
to <emphasis>return</emphasis> the value, not <function>echo</function>
|
|
it.
|
|
</para>
|
|
</answer>
|
|
</qandaentry>
|
|
|
|
<qandaentry id="faq.using.newlines">
|
|
<question>
|
|
<para>
|
|
Hey, what happened to my newlines?
|
|
<programlisting role="php">
|
|
<![CDATA[
|
|
<pre>
|
|
<?php echo "This should be the first line."; ?>
|
|
<?php echo "This should show up after the new line above."; ?>
|
|
</pre>
|
|
]]>
|
|
</programlisting>
|
|
</para>
|
|
</question>
|
|
<answer>
|
|
<para>
|
|
In PHP, the ending for a block of code is either "?>" or
|
|
"?>\n" (where \n means a newline). So in the example above,
|
|
the echoed sentences will be on one line, because PHP ommits
|
|
the newlines after the block ending. This means that you need to
|
|
insert an extra newline after each block of PHP code to make
|
|
it print out one newline.
|
|
</para>
|
|
<para>
|
|
Why does PHP do this? Because when formatting normal HTML, this
|
|
usually makes your life easier because you don't want that newline,
|
|
but you'd have to create extremely long lines or otherwise make the
|
|
raw page source unreadable to achieve that effect.
|
|
</para>
|
|
</answer>
|
|
</qandaentry>
|
|
|
|
<qandaentry id="faq.using.header">
|
|
<question>
|
|
<para>
|
|
I need to access information in the request header directly.
|
|
How can I do this?
|
|
</para>
|
|
</question>
|
|
<answer>
|
|
<para>
|
|
The <function>getallheaders</function> function will do this if
|
|
you are running PHP as an Apache module. So, the following bit
|
|
of code will show you all the request headers:
|
|
<programlisting role="php">
|
|
<![CDATA[
|
|
$headers = getallheaders();
|
|
foreach ($headers as $name => $content) {
|
|
echo "headers[$name] = $content<br>\n";
|
|
}
|
|
]]>
|
|
</programlisting>
|
|
</para>
|
|
</answer>
|
|
</qandaentry>
|
|
|
|
<qandaentry id="faq.using.authentication">
|
|
<question>
|
|
<para>
|
|
When I try to use authentication with IIS I get 'No Input file specified'.
|
|
</para>
|
|
</question>
|
|
<answer>
|
|
<para>
|
|
The security model of IIS is at fault here. This is a problem
|
|
common to all CGI programs running under IIS. A workaround is
|
|
to create a plain HTML file (not parsed by PHP) as the entry page
|
|
into an authenticated directory. Then use a META tag to redirect
|
|
to the PHP page, or have a link to the PHP page. PHP will
|
|
then recognize the authentication correctly. With the ISAPI
|
|
module, this is not a problem. This should not effect other
|
|
NT web servers. For more information, see:
|
|
<ulink url="&faqurl.iis;">&faqurl.iis;</ulink>.
|
|
</para>
|
|
</answer>
|
|
</qandaentry>
|
|
|
|
<qandaentry id="faq.using.netscape">
|
|
<question>
|
|
<para>
|
|
My PHP script works on IE and Lynx, but on Netscape some of
|
|
my output is missing. When I do a "View Source" I see the
|
|
content in IE but not in Netscape.
|
|
</para>
|
|
</question>
|
|
<answer>
|
|
<para>
|
|
Netscape is more strict regarding html tags (such as tables) then
|
|
IE. Running your html output through a html validator, such as
|
|
<ulink url="&faqurl.w3.validator;">validator.w3.org</ulink>, might
|
|
be helpful. For example, a missing </table> might cause this.
|
|
</para>
|
|
<para>
|
|
Also, both IE and Lynx ignore any NULs (<literal>\0</literal>) in
|
|
the HTML stream, Netscape does not. The best way to check for this is
|
|
to compile the <link linkend="commandline">command line</link> version of
|
|
PHP (also known as the CGI version) and run your script from the
|
|
command line. In *nix, pipe it through <literal>od -c</literal> and look
|
|
for any <literal>\0</literal> characters. If you are on Windows you need
|
|
to find an editor or some other program that lets you look at binary files.
|
|
When Netscape sees a NUL in a file it will typically not output anything
|
|
else on that line whereas both IE and Lynx will.
|
|
</para>
|
|
</answer>
|
|
</qandaentry>
|
|
|
|
<qandaentry id="faq.using.mixml">
|
|
<question>
|
|
<para>
|
|
How am I supposed to mix XML and PHP? It complains
|
|
about my <?xml> tags!
|
|
</para>
|
|
</question>
|
|
<answer>
|
|
<para>
|
|
You need to turn off the short tags by setting
|
|
<link linkend="ini.short-open-tag">short_tags</link> to 0 in your
|
|
<filename>php.ini</filename> file, or by using the appropriate
|
|
Apache directive. You could even use a <File> section to
|
|
do this selectively.
|
|
</para>
|
|
</answer>
|
|
</qandaentry>
|
|
|
|
<qandaentry id="faq.using.editor">
|
|
<question>
|
|
<para>
|
|
How can I use PHP with FrontPage or some other HTML editor
|
|
that insists on moving my code around?
|
|
</para>
|
|
</question>
|
|
<answer>
|
|
<para>
|
|
One of the easiest things to do is to enable using ASP tags in your
|
|
PHP code. This allows you to use the ASP-style <% and %> code
|
|
delimiters. Some of the popular HTML editors handle those more
|
|
intelligently (for now). To enable the ASP-style tags, you need
|
|
to set the <link linkend="ini.asp-tags">asp_tags</link>
|
|
<filename>php.ini</filename> variable, or use the
|
|
appropriate Apache directive.
|
|
</para>
|
|
</answer>
|
|
</qandaentry>
|
|
|
|
<qandaentry id="faq.using.variables">
|
|
<question>
|
|
<para>
|
|
Where can I find a complete list of pre-set variables available
|
|
to me, and why are these not documented in the PHP documentation?
|
|
</para>
|
|
</question>
|
|
<answer>
|
|
<para>
|
|
The best way is to stick a <literal><?php phpinfo(); ?></literal>
|
|
part on a page and load it up. This will show you all sorts of
|
|
information about your PHP setup, including a list of both
|
|
environment variables and also special variables set by your
|
|
web server. This list can't really be documented in the PHP
|
|
documentation because it will change from one server to another.
|
|
</para>
|
|
</answer>
|
|
</qandaentry>
|
|
|
|
<qandaentry id="faq.using.cgi-vars">
|
|
<question>
|
|
<para>
|
|
I'm trying to access one of the standard CGI
|
|
variables (such as $DOCUMENT_ROOT or $HTTP_REFERER) in a user-defined
|
|
function, and it can't seem to find it. What's wrong?
|
|
</para>
|
|
</question>
|
|
<answer>
|
|
<para>
|
|
Environment variables are normal global variables, so you must
|
|
either declare them as global variables in your function (by using
|
|
"<literal>global $DOCUMENT_ROOT;</literal>", for example) or by using
|
|
the global variable array (ie, "<literal>$GLOBALS["DOCUMENT_ROOT"]</literal>".
|
|
</para>
|
|
</answer>
|
|
</qandaentry>
|
|
|
|
</qandaset>
|
|
</chapter>
|
|
|
|
<!-- Keep this comment at the end of the file
|
|
Local variables:
|
|
mode: sgml
|
|
sgml-omittag:t
|
|
sgml-shorttag:t
|
|
sgml-minimize-attributes:nil
|
|
sgml-always-quote-attributes:t
|
|
sgml-indent-step:1
|
|
sgml-indent-data:t
|
|
sgml-parent-document:nil
|
|
sgml-default-dtd-file:"../../manual.ced"
|
|
sgml-exposed-tags:nil
|
|
sgml-local-catalogs:nil
|
|
sgml-local-ecat-files:nil
|
|
End:
|
|
vim600: syn=xml fen fdm=syntax fdl=2 si
|
|
vim: et tw=78 syn=sgml
|
|
vi: ts=1 sw=1
|
|
-->
|