mirror of
https://github.com/sigmasternchen/php-doc-en
synced 2025-03-19 10:28:54 +00:00
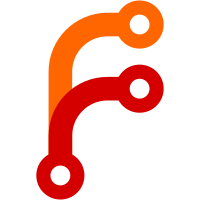
git-svn-id: https://svn.php.net/repository/phpdoc/en/trunk@322055 c90b9560-bf6c-de11-be94-00142212c4b1
389 lines
11 KiB
XML
389 lines
11 KiB
XML
<?xml version="1.0" encoding="utf-8"?>
|
|
<!-- $Revision$ -->
|
|
<refentry xmlns="http://docbook.org/ns/docbook" xml:id="function.maxdb-stmt-bind-param">
|
|
<refnamediv>
|
|
<refname>maxdb_stmt_bind_param</refname>
|
|
<refname>maxdb_stmt::bind_param</refname>
|
|
<refpurpose>Binds variables to a prepared statement as parameters</refpurpose>
|
|
</refnamediv>
|
|
|
|
<refsect1 role="description">
|
|
&reftitle.description;
|
|
<para>&style.procedural;</para>
|
|
<methodsynopsis>
|
|
<type>bool</type><methodname>maxdb_stmt_bind_param</methodname>
|
|
<methodparam><type>resource</type><parameter>stmt</parameter></methodparam>
|
|
<methodparam><type>string</type><parameter>types</parameter></methodparam>
|
|
<methodparam><type>mixed</type><parameter role="reference">var1</parameter></methodparam>
|
|
<methodparam choice="opt"><type>mixed</type><parameter role="reference">...</parameter></methodparam>
|
|
</methodsynopsis>
|
|
<para>&style.oop;</para>
|
|
<methodsynopsis>
|
|
<type>bool</type><methodname>maxdb_stmt::bind_param</methodname>
|
|
<methodparam><type>string</type><parameter>types</parameter></methodparam>
|
|
<methodparam><type>mixed</type><parameter role="reference">var1</parameter></methodparam>
|
|
<methodparam choice="opt"><type>mixed</type><parameter role="reference">...</parameter></methodparam>
|
|
</methodsynopsis>
|
|
<para>&style.procedural; (extended syntax):</para>
|
|
<methodsynopsis>
|
|
<type>bool</type><methodname>maxdb_stmt_bind_param</methodname>
|
|
<methodparam><type>resource</type><parameter>stmt</parameter></methodparam>
|
|
<methodparam><type>string</type><parameter>types</parameter></methodparam>
|
|
<methodparam><type>array</type><parameter role="reference">var</parameter></methodparam>
|
|
</methodsynopsis>
|
|
<para>&style.oop; (extended syntax):</para>
|
|
<methodsynopsis>
|
|
<type>bool</type><methodname>maxdb_stmt::bind_param</methodname>
|
|
<methodparam><type>string</type><parameter>types</parameter></methodparam>
|
|
<methodparam><type>array</type><parameter role="reference">var</parameter></methodparam>
|
|
</methodsynopsis>
|
|
<para>
|
|
<function>maxdb_stmt_bind_param</function> is used to bind variables for the
|
|
parameter markers in the SQL statement that was passed to
|
|
<function>maxdb_prepare</function>.
|
|
The string <parameter>types</parameter> contains one or more characters which specify
|
|
the types for the corresponding bind variables.
|
|
</para>
|
|
<para>
|
|
The extended syntax of <function>maxdb_stmt_bind_param</function> allows to give the parameters
|
|
as an array instead of a variable list of PHP variables to the function. If the array variable has not been
|
|
used before calling <function>maxdb_stmt_bind_param</function>, it has to be initialized as an emtpy
|
|
array. See the examples how to use <function>maxdb_stmt_bind_param</function> with extended syntax.
|
|
</para>
|
|
<para>
|
|
Variables for SELECT INTO SQL statements can also be bound using <function>maxdb_stmt_bind_param</function>.
|
|
Parameters for database procedures can be bound using <function>maxdb_stmt_bind_param</function>. See the
|
|
examples how to use <function>maxdb_stmt_bind_param</function> in this cases.
|
|
</para>
|
|
<para>
|
|
If a variable bound as INTO variable to an SQL statement was used before, the content of this variable
|
|
is overwritten by the data of the SELECT INTO statement. A reference to this variable will be invalid after a call to
|
|
<function>maxdb_stmt_bind_param</function>.
|
|
</para>
|
|
<para>
|
|
For INOUT parameters of database procedures the content of the bound INOUT variable is overwritten by the output
|
|
value of the database procedure. A reference to this variable will be invalid after a call to
|
|
<function>maxdb_stmt_bind_param</function>.
|
|
</para>
|
|
<para>
|
|
<table>
|
|
<title>Type specification chars</title>
|
|
<tgroup cols="2">
|
|
<thead>
|
|
<row>
|
|
<entry>Character</entry>
|
|
<entry>Description</entry>
|
|
</row>
|
|
</thead>
|
|
<tbody>
|
|
<row>
|
|
<entry>i</entry>
|
|
<entry>corresponding variable has type integer</entry>
|
|
</row>
|
|
<row>
|
|
<entry>d</entry>
|
|
<entry>corresponding variable has type double</entry>
|
|
</row>
|
|
<row>
|
|
<entry>s</entry>
|
|
<entry>corresponding variable has type string</entry>
|
|
</row>
|
|
<row>
|
|
<entry>b</entry>
|
|
<entry>corresponding variable is a blob and will be sent in packages</entry>
|
|
</row>
|
|
</tbody>
|
|
</tgroup>
|
|
</table>
|
|
</para>
|
|
</refsect1>
|
|
|
|
<refsect1 role="returnvalues">
|
|
&reftitle.returnvalues;
|
|
<para>
|
|
&return.success;
|
|
</para>
|
|
</refsect1>
|
|
|
|
<refsect1 role="examples">
|
|
&reftitle.examples;
|
|
<example>
|
|
<title>&style.oop;</title>
|
|
<programlisting role="php">
|
|
<![CDATA[
|
|
<?php
|
|
$maxdb = new maxdb('localhost', 'MONA', 'RED', 'DEMODB');
|
|
|
|
/* check connection */
|
|
if (maxdb_connect_errno()) {
|
|
printf("Connect failed: %s\n", maxdb_connect_error());
|
|
exit();
|
|
}
|
|
|
|
$maxdb->query ("CREATE TABLE temp.mycity LIKE hotel.city");
|
|
$maxdb->query ("INSERT INTO temp.mycity SELECT * FROM hotel.city");
|
|
|
|
$stmt = $maxdb->prepare("INSERT INTO temp.mycity VALUES (?, ?, ?)");
|
|
$stmt->bind_param('sss', $zip, $name, $state);
|
|
|
|
$zip = '11111';
|
|
$name = 'Georgetown';
|
|
$state = 'NY';
|
|
|
|
/* execute prepared statement */
|
|
$stmt->execute();
|
|
|
|
printf("%d Row inserted.\n", $stmt->affected_rows);
|
|
|
|
/* close statement and connection */
|
|
$stmt->close();
|
|
|
|
/* Clean up table CountryLanguage */
|
|
$maxdb->query("DELETE FROM temp.mycity WHERE name='Georgetown'");
|
|
printf("%d Row deleted.\n", $maxdb->affected_rows);
|
|
|
|
/* close connection */
|
|
$maxdb->close();
|
|
?>
|
|
]]>
|
|
</programlisting>
|
|
</example>
|
|
<example>
|
|
<title>&style.procedural;</title>
|
|
<programlisting role="php">
|
|
<![CDATA[
|
|
<?php
|
|
$link = maxdb_connect("localhost", "MONA", "RED", "DEMODB");
|
|
|
|
/* check connection */
|
|
if (!$link) {
|
|
printf("Connect failed: %s\n", maxdb_connect_error());
|
|
exit();
|
|
}
|
|
|
|
maxdb_query ($link, "CREATE TABLE temp.mycity LIKE hotel.city");
|
|
maxdb_query ($link, "INSERT INTO temp.mycity SELECT * FROM hotel.city");
|
|
|
|
$stmt = maxdb_prepare($link, "INSERT INTO temp.mycity VALUES (?, ?, ?)");
|
|
maxdb_stmt_bind_param($stmt, 'sss', $zip, $name, $state);
|
|
|
|
$zip = '11111';
|
|
$name = 'Georgetown';
|
|
$state = 'NY';
|
|
|
|
/* execute prepared statement */
|
|
maxdb_stmt_execute($stmt);
|
|
|
|
printf("%d Row inserted.\n", maxdb_stmt_affected_rows($stmt));
|
|
|
|
/* close statement and connection */
|
|
maxdb_stmt_close($stmt);
|
|
|
|
/* Clean up table CountryLanguage */
|
|
maxdb_query($link, "DELETE FROM temp.mycity WHERE name='Georgetown'");
|
|
printf("%d Row deleted.\n", maxdb_affected_rows($link));
|
|
|
|
/* close connection */
|
|
maxdb_close($link);
|
|
?>
|
|
]]>
|
|
</programlisting>
|
|
</example>
|
|
&example.outputs.similar;
|
|
<screen>
|
|
<![CDATA[
|
|
1 Row inserted.
|
|
1 Row deleted.
|
|
]]>
|
|
</screen>
|
|
<example>
|
|
<title>&style.procedural; (SELECT INTO)</title>
|
|
<programlisting role="php">
|
|
<![CDATA[
|
|
<?php
|
|
$link = maxdb_connect("localhost", "MONA", "RED", "DEMODB");
|
|
|
|
/* check connection */
|
|
if (!$link) {
|
|
printf("Connect failed: %s\n", maxdb_connect_error());
|
|
exit();
|
|
}
|
|
|
|
/* Performing SQL query */
|
|
$stmt = maxdb_prepare ($link, "SELECT price INTO ? FROM hotel.room where hno = ? and type = ?");
|
|
if (!$stmt) {
|
|
printf ("Prepare failed: %s\n", maxdb_error($link));
|
|
}
|
|
|
|
$hno = "50";
|
|
$rtype = "suite";
|
|
|
|
maxdb_stmt_bind_param($stmt, 'dss', $price, $hno, $rtype);
|
|
maxdb_stmt_execute($stmt);
|
|
|
|
printf ("%f\n", $price);
|
|
|
|
maxdb_stmt_close ($stmt);
|
|
?>
|
|
]]>
|
|
</programlisting>
|
|
</example>
|
|
&example.outputs.similar;
|
|
<screen>
|
|
<![CDATA[
|
|
21.600000
|
|
]]>
|
|
</screen>
|
|
<example>
|
|
<title>&style.procedural; (DB procedure)</title>
|
|
<programlisting role="php">
|
|
<![CDATA[
|
|
<?php
|
|
$link = maxdb_connect("localhost", "MONA", "RED", "DEMODB");
|
|
|
|
/* check connection */
|
|
if (!$link) {
|
|
printf("Connect failed: %s\n", maxdb_connect_error());
|
|
exit();
|
|
}
|
|
|
|
maxdb_report (MAXDB_REPORT_OFF);
|
|
maxdb_query($link,"DROP DBPROC test_proc");
|
|
maxdb_report (MAXDB_REPORT_ERROR);
|
|
|
|
$query = "create dbproc test_proc (INOUT e_text char(72)) AS select * from SYSDBA.DUAL; fetch into :e_text;";
|
|
|
|
maxdb_query($link, $query);
|
|
|
|
/* Performing SQL query */
|
|
$stmt = maxdb_prepare ($link, "CALL test_proc (?)");
|
|
if (!$stmt) {
|
|
printf ("Prepare failed: %s\n", maxdb_error($link));
|
|
}
|
|
|
|
maxdb_stmt_bind_param($stmt, 's', $result);
|
|
maxdb_stmt_execute($stmt);
|
|
|
|
printf ("%s\n", $result);
|
|
|
|
maxdb_stmt_close ($stmt);
|
|
?>
|
|
]]>
|
|
</programlisting>
|
|
</example>
|
|
&example.outputs.similar;
|
|
<screen>
|
|
<![CDATA[
|
|
a
|
|
]]>
|
|
</screen>
|
|
<example>
|
|
<title>&style.oop; (extended syntax)</title>
|
|
<programlisting role="php">
|
|
<![CDATA[
|
|
<?php
|
|
$maxdb = new maxdb('localhost', 'MONA', 'RED', 'DEMODB');
|
|
|
|
/* check connection */
|
|
if (maxdb_connect_errno()) {
|
|
printf("Connect failed: %s\n", maxdb_connect_error());
|
|
exit();
|
|
}
|
|
|
|
$maxdb->query ("CREATE TABLE temp.mycity LIKE hotel.city");
|
|
$maxdb->query ("INSERT INTO temp.mycity SELECT * FROM hotel.city");
|
|
|
|
$stmt = $maxdb->prepare("INSERT INTO temp.mycity VALUES (?, ?, ?)");
|
|
|
|
$arr = array();
|
|
|
|
$stmt->bind_param('iss', $arr);
|
|
|
|
$arr[0] = 11111;
|
|
$arr[1] = 'Georgetown';
|
|
$arr[2] = 'NY';
|
|
|
|
/* execute prepared statement */
|
|
$stmt->execute();
|
|
|
|
printf("%d Row inserted.\n", maxdb_stmt_affected_rows($stmt));
|
|
|
|
$arr[0] = 22222;
|
|
$arr[1] = 'New Orleans';
|
|
$arr[2] = 'LA';
|
|
|
|
/* execute prepared statement */
|
|
$stmt->execute();
|
|
|
|
printf("%d Row inserted.\n", $stmt->affected_rows);
|
|
|
|
/* close statement and connection */
|
|
$stmt->close();
|
|
|
|
$result = $maxdb->query("SELECT * from temp.mycity WHERE zip = '11111' OR zip = '22222'");
|
|
if ($result) {
|
|
while ($row = $result->fetch_row()) {
|
|
printf ("%s %s %s\n", $row[0], $row[1], $row[2]);
|
|
}
|
|
}
|
|
|
|
/* Clean up table CountryLanguage */
|
|
$maxdb->query("DELETE FROM temp.mycity WHERE name='Georgetown'");
|
|
$maxdb->query("DELETE FROM temp.mycity WHERE name='New Orleans'");
|
|
printf("%d Rows deleted.\n", $maxdb->affected_rows);
|
|
|
|
/* close connection */
|
|
$maxdb->close();
|
|
?>
|
|
]]>
|
|
</programlisting>
|
|
</example>
|
|
&example.outputs.similar;
|
|
<screen>
|
|
<![CDATA[
|
|
1 Row inserted.
|
|
1 Row inserted.
|
|
11111 Georgetown NY
|
|
22222 New Orleans LA
|
|
2 Rows deleted.
|
|
]]>
|
|
</screen>
|
|
</refsect1>
|
|
|
|
<refsect1 role="seealso">
|
|
&reftitle.seealso;
|
|
<para>
|
|
<simplelist>
|
|
<member><function>maxdb_stmt_bind_result</function></member>
|
|
<member><function>maxdb_stmt_execute</function></member>
|
|
<member><function>maxdb_stmt_fetch</function></member>
|
|
<member><function>maxdb_prepare</function></member>
|
|
<member><function>maxdb_stmt_send_long_data</function></member>
|
|
<member><function>maxdb_stmt_errno</function></member>
|
|
<member><function>maxdb_stmt_error</function></member>
|
|
</simplelist>
|
|
</para>
|
|
</refsect1>
|
|
|
|
</refentry>
|
|
|
|
<!-- Keep this comment at the end of the file
|
|
Local variables:
|
|
mode: sgml
|
|
sgml-omittag:t
|
|
sgml-shorttag:t
|
|
sgml-minimize-attributes:nil
|
|
sgml-always-quote-attributes:t
|
|
sgml-indent-step:1
|
|
sgml-indent-data:t
|
|
indent-tabs-mode:nil
|
|
sgml-parent-document:nil
|
|
sgml-default-dtd-file:"~/.phpdoc/manual.ced"
|
|
sgml-exposed-tags:nil
|
|
sgml-local-catalogs:nil
|
|
sgml-local-ecat-files:nil
|
|
End:
|
|
vim600: syn=xml fen fdm=syntax fdl=2 si
|
|
vim: et tw=78 syn=sgml
|
|
vi: ts=1 sw=1
|
|
-->
|