mirror of
https://github.com/sigmasternchen/php-doc-en
synced 2025-03-15 16:38:54 +00:00
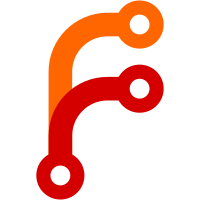
git-svn-id: https://svn.php.net/repository/phpdoc/en/trunk@297028 c90b9560-bf6c-de11-be94-00142212c4b1
446 lines
9.1 KiB
XML
446 lines
9.1 KiB
XML
<?xml version="1.0" encoding="utf-8"?>
|
|
<!-- $Revision$ -->
|
|
|
|
<chapter xml:id="ming.examples" xmlns="http://docbook.org/ns/docbook">
|
|
&reftitle.examples;
|
|
<section xml:id="ming.examples.swfaction">
|
|
<title>SWFAction Examples</title>
|
|
<para>
|
|
This simple example will move the red square across the window.
|
|
<example>
|
|
<title><function>swfaction</function> example</title>
|
|
<programlisting role="php">
|
|
<![CDATA[
|
|
<?php
|
|
$s = new SWFShape();
|
|
$f = $s->addFill(0xff, 0, 0);
|
|
$s->setRightFill($f);
|
|
|
|
$s->movePenTo(-500, -500);
|
|
$s->drawLineTo(500, -500);
|
|
$s->drawLineTo(500, 500);
|
|
$s->drawLineTo(-500, 500);
|
|
$s->drawLineTo(-500, -500);
|
|
|
|
$p = new SWFSprite();
|
|
$i = $p->add($s);
|
|
$i->setDepth(1);
|
|
$p->nextFrame();
|
|
|
|
for ($n=0; $n<5; ++$n) {
|
|
$i->rotate(-15);
|
|
$p->nextFrame();
|
|
}
|
|
|
|
$m = new SWFMovie();
|
|
$m->setBackground(0xff, 0xff, 0xff);
|
|
$m->setDimension(6000, 4000);
|
|
|
|
$i = $m->add($p);
|
|
$i->setDepth(1);
|
|
$i->moveTo(-500,2000);
|
|
$i->setName("box");
|
|
|
|
$m->add(new SWFAction("/box.x += 3;"));
|
|
$m->nextFrame();
|
|
$m->add(new SWFAction("gotoFrame(0); play();"));
|
|
$m->nextFrame();
|
|
|
|
header('Content-type: application/x-shockwave-flash');
|
|
$m->output();
|
|
?>
|
|
]]>
|
|
</programlisting>
|
|
</example>
|
|
</para>
|
|
<para>
|
|
This simple example tracks down your mouse on the screen.
|
|
<example>
|
|
<title><function>swfaction</function> example</title>
|
|
<programlisting role="php">
|
|
<![CDATA[
|
|
<?php
|
|
|
|
$m = new SWFMovie();
|
|
$m->setRate(36.0);
|
|
$m->setDimension(1200, 800);
|
|
$m->setBackground(0, 0, 0);
|
|
|
|
/* mouse tracking sprite - empty, but follows mouse so we can
|
|
get its x and y coordinates */
|
|
|
|
$i = $m->add(new SWFSprite());
|
|
$i->setName('mouse');
|
|
|
|
$m->add(new SWFAction("
|
|
startDrag('/mouse', 1); /* '1' means lock sprite to the mouse */
|
|
"));
|
|
|
|
/* might as well turn off antialiasing, since these are just squares. */
|
|
|
|
$m->add(new SWFAction("
|
|
this.quality = 0;
|
|
"));
|
|
|
|
/* morphing box */
|
|
$r = new SWFMorph();
|
|
$s = $r->getShape1();
|
|
|
|
/* Note this is backwards from normal shapes. No idea why. */
|
|
$s->setLeftFill($s->addFill(0xff, 0xff, 0xff));
|
|
$s->movePenTo(-40, -40);
|
|
$s->drawLine(80, 0);
|
|
$s->drawLine(0, 80);
|
|
$s->drawLine(-80, 0);
|
|
$s->drawLine(0, -80);
|
|
|
|
$s = $r->getShape2();
|
|
|
|
$s->setLeftFill($s->addFill(0x00, 0x00, 0x00));
|
|
$s->movePenTo(-1, -1);
|
|
$s->drawLine(2, 0);
|
|
$s->drawLine(0, 2);
|
|
$s->drawLine(-2, 0);
|
|
$s->drawLine(0, -2);
|
|
|
|
/* sprite container for morphing box -
|
|
this is just a timeline w/ the box morphing */
|
|
|
|
$box = new SWFSprite();
|
|
$box->add(new SWFAction("
|
|
stop();
|
|
"));
|
|
$i = $box->add($r);
|
|
|
|
for ($n=0; $n<=20; ++$n) {
|
|
$i->setRatio($n/20);
|
|
$box->nextFrame();
|
|
}
|
|
|
|
/* this container sprite allows us to use the same action code many times */
|
|
|
|
$cell = new SWFSprite();
|
|
$i = $cell->add($box);
|
|
$i->setName('box');
|
|
|
|
$cell->add(new SWFAction("
|
|
|
|
setTarget('box');
|
|
|
|
/* ...x means the x coordinate of the parent, i.e. (..).x */
|
|
dx = (/mouse.x + random(6)-3 - ...x)/5;
|
|
dy = (/mouse.y + random(6)-3 - ...y)/5;
|
|
gotoFrame(int(dx*dx + dy*dy));
|
|
|
|
"));
|
|
|
|
$cell->nextFrame();
|
|
$cell->add(new SWFAction("
|
|
|
|
gotoFrame(0);
|
|
play();
|
|
|
|
"));
|
|
|
|
$cell->nextFrame();
|
|
|
|
/* finally, add a bunch of the cells to the movie */
|
|
|
|
for ($x=0; $x<12; ++$x) {
|
|
for ($y=0; $y<8; ++$y) {
|
|
$i = $m->add($cell);
|
|
$i->moveTo(100*$x+50, 100*$y+50);
|
|
}
|
|
}
|
|
|
|
$m->nextFrame();
|
|
|
|
$m->add(new SWFAction("
|
|
|
|
gotoFrame(1);
|
|
play();
|
|
|
|
"));
|
|
|
|
header('Content-type: application/x-shockwave-flash');
|
|
$m->output();
|
|
?>
|
|
]]>
|
|
</programlisting>
|
|
</example>
|
|
</para>
|
|
<para>
|
|
Same as above, but with nice colored balls...
|
|
<example>
|
|
<title><function>swfaction</function> example</title>
|
|
<programlisting role="php">
|
|
<![CDATA[
|
|
<?php
|
|
|
|
$m = new SWFMovie();
|
|
$m->setDimension(11000, 8000);
|
|
$m->setBackground(0x00, 0x00, 0x00);
|
|
|
|
$m->add(new SWFAction("
|
|
|
|
this.quality = 0;
|
|
/frames.visible = 0;
|
|
startDrag('/mouse', 1);
|
|
|
|
"));
|
|
|
|
// mouse tracking sprite
|
|
$t = new SWFSprite();
|
|
$i = $m->add($t);
|
|
$i->setName('mouse');
|
|
|
|
$g = new SWFGradient();
|
|
$g->addEntry(0, 0xff, 0xff, 0xff, 0xff);
|
|
$g->addEntry(0.1, 0xff, 0xff, 0xff, 0xff);
|
|
$g->addEntry(0.5, 0xff, 0xff, 0xff, 0x5f);
|
|
$g->addEntry(1.0, 0xff, 0xff, 0xff, 0);
|
|
|
|
// gradient shape thing
|
|
$s = new SWFShape();
|
|
$f = $s->addFill($g, SWFFILL_RADIAL_GRADIENT);
|
|
$f->scaleTo(0.03);
|
|
$s->setRightFill($f);
|
|
$s->movePenTo(-600, -600);
|
|
$s->drawLine(1200, 0);
|
|
$s->drawLine(0, 1200);
|
|
$s->drawLine(-1200, 0);
|
|
$s->drawLine(0, -1200);
|
|
|
|
// need to make this a sprite so we can multColor it
|
|
$p = new SWFSprite();
|
|
$p->add($s);
|
|
$p->nextFrame();
|
|
|
|
// put the shape in here, each frame a different color
|
|
$q = new SWFSprite();
|
|
$q->add(new SWFAction("gotoFrame(random(7)+1); stop();"));
|
|
$i = $q->add($p);
|
|
|
|
$i->multColor(1.0, 1.0, 1.0);
|
|
$q->nextFrame();
|
|
$i->multColor(1.0, 0.5, 0.5);
|
|
$q->nextFrame();
|
|
$i->multColor(1.0, 0.75, 0.5);
|
|
$q->nextFrame();
|
|
$i->multColor(1.0, 1.0, 0.5);
|
|
$q->nextFrame();
|
|
$i->multColor(0.5, 1.0, 0.5);
|
|
$q->nextFrame();
|
|
$i->multColor(0.5, 0.5, 1.0);
|
|
$q->nextFrame();
|
|
$i->multColor(1.0, 0.5, 1.0);
|
|
$q->nextFrame();
|
|
|
|
// finally, this one contains the action code
|
|
$p = new SWFSprite();
|
|
$i = $p->add($q);
|
|
$i->setName('frames');
|
|
$p->add(new SWFAction("
|
|
|
|
dx = (/:mousex-/:lastx)/3 + random(10)-5;
|
|
dy = (/:mousey-/:lasty)/3;
|
|
x = /:mousex;
|
|
y = /:mousey;
|
|
alpha = 100;
|
|
|
|
"));
|
|
$p->nextFrame();
|
|
|
|
$p->add(new SWFAction("
|
|
|
|
this.x = x;
|
|
this.y = y;
|
|
this.alpha = alpha;
|
|
x += dx;
|
|
y += dy;
|
|
dy += 3;
|
|
alpha -= 8;
|
|
|
|
"));
|
|
$p->nextFrame();
|
|
|
|
$p->add(new SWFAction("prevFrame(); play();"));
|
|
$p->nextFrame();
|
|
|
|
$i = $m->add($p);
|
|
$i->setName('frames');
|
|
$m->nextFrame();
|
|
|
|
$m->add(new SWFAction("
|
|
|
|
lastx = mousex;
|
|
lasty = mousey;
|
|
mousex = /mouse.x;
|
|
mousey = /mouse.y;
|
|
|
|
++num;
|
|
|
|
if (num == 11)
|
|
num = 1;
|
|
|
|
removeClip('char' & num);
|
|
duplicateClip(/frames, 'char' & num, num);
|
|
|
|
"));
|
|
|
|
$m->nextFrame();
|
|
$m->add(new SWFAction("prevFrame(); play();"));
|
|
|
|
header('Content-type: application/x-shockwave-flash');
|
|
$m->output();
|
|
?>
|
|
]]>
|
|
</programlisting>
|
|
</example>
|
|
</para>
|
|
<!-- FIXME: Serious syntax errors in example
|
|
<para>
|
|
This simple example will handles keyboard actions.
|
|
(You'll probably have to click in the window to give it focus.
|
|
And you'll probably have to leave your mouse in the frame, too.
|
|
If you know how to give buttons focus programatically, feel free
|
|
to share, won't you?)
|
|
<example>
|
|
<title><function>swfaction</function> example</title>
|
|
<programlisting role="php">
|
|
<![CDATA[
|
|
<?php
|
|
|
|
/* sprite has one letter per frame */
|
|
|
|
$p = new SWFSprite();
|
|
$p->add(new SWFAction("stop();"));
|
|
|
|
$chars = 'abcdefghijklmnopqrstuvwxyz'.
|
|
'ABCDEFGHIJKLMNOPQRSTUVWXYZ'.
|
|
'1234567890!@#$%^&/*()_+-=/[]{}|;:,.<>?`~';
|
|
|
|
$f = new SWFFont("_sans");
|
|
|
|
for ($n=0; $nremove($i);
|
|
$t = new SWFTextField();
|
|
$t->setFont($f);
|
|
$t->setHeight(240);
|
|
$t->setBounds(600,240);
|
|
$t->align(SWFTEXTFIELD_ALIGN_CENTER);
|
|
$t->addString($c);
|
|
$i = $p->add($t);
|
|
$p->labelFrame($c);
|
|
$p->nextFrame();
|
|
}
|
|
|
|
/* hit region for button - the entire frame */
|
|
|
|
$s = new SWFShape();
|
|
$s->setFillStyle0($s->addSolidFill(0, 0, 0, 0));
|
|
$s->drawLine(600, 0);
|
|
$s->drawLine(0, 400);
|
|
$s->drawLine(-600, 0);
|
|
$s->drawLine(0, -400);
|
|
|
|
/* button checks for pressed key, sends sprite to the right frame */
|
|
|
|
$b = new SWFButton();
|
|
$b->addShape($s, SWFBUTTON_HIT);
|
|
|
|
for($n=0; $naddAction(new SWFAction("
|
|
|
|
setTarget('/char');
|
|
gotoFrame('$c');
|
|
|
|
"), SWFBUTTON_KEYPRESS($c));
|
|
}
|
|
|
|
$m = new SWFMovie();
|
|
$m->setDimension(600,400);
|
|
$i = $m->add($p);
|
|
$i->setName('char');
|
|
$i->moveTo(0,80);
|
|
|
|
$m->add($b);
|
|
|
|
header('Content-type: application/x-shockwave-flash');
|
|
$m->output();
|
|
|
|
?>
|
|
]]>
|
|
</programlisting>
|
|
</example>
|
|
</para>
|
|
-->
|
|
</section>
|
|
<section xml:id="ming.examples.swfsprite-basic">
|
|
<title>SWFSPrite basic examples</title>
|
|
<para>
|
|
This simple example will spin gracefully a big red square.
|
|
<example>
|
|
<title><function>swfsprite</function> example</title>
|
|
<programlisting role="php">
|
|
<![CDATA[
|
|
<?php
|
|
$s = new SWFShape();
|
|
$s->setRightFill($s->addFill(0xff, 0, 0));
|
|
$s->movePenTo(-500, -500);
|
|
$s->drawLineTo(500, -500);
|
|
$s->drawLineTo(500, 500);
|
|
$s->drawLineTo(-500, 500);
|
|
$s->drawLineTo(-500, -500);
|
|
|
|
$p = new SWFSprite();
|
|
$i = $p->add($s);
|
|
$p->nextFrame();
|
|
$i->rotate(15);
|
|
$p->nextFrame();
|
|
$i->rotate(15);
|
|
$p->nextFrame();
|
|
$i->rotate(15);
|
|
$p->nextFrame();
|
|
$i->rotate(15);
|
|
$p->nextFrame();
|
|
$i->rotate(15);
|
|
$p->nextFrame();
|
|
|
|
$m = new SWFMovie();
|
|
$i = $m->add($p);
|
|
$i->moveTo(1500, 1000);
|
|
$i->setName("blah");
|
|
|
|
$m->setBackground(0xff, 0xff, 0xff);
|
|
$m->setDimension(3000, 2000);
|
|
|
|
header('Content-type: application/x-shockwave-flash');
|
|
$m->output();
|
|
?>
|
|
]]>
|
|
</programlisting>
|
|
</example>
|
|
</para>
|
|
</section>
|
|
|
|
</chapter>
|
|
|
|
<!-- Keep this comment at the end of the file
|
|
Local variables:
|
|
mode: sgml
|
|
sgml-omittag:t
|
|
sgml-shorttag:t
|
|
sgml-minimize-attributes:nil
|
|
sgml-always-quote-attributes:t
|
|
sgml-indent-step:1
|
|
sgml-indent-data:t
|
|
indent-tabs-mode:nil
|
|
sgml-parent-document:nil
|
|
sgml-default-dtd-file:"~/.phpdoc/manual.ced"
|
|
sgml-exposed-tags:nil
|
|
sgml-local-catalogs:nil
|
|
sgml-local-ecat-files:nil
|
|
End:
|
|
vim600: syn=xml fen fdm=syntax fdl=2 si
|
|
vim: et tw=78 syn=sgml
|
|
vi: ts=1 sw=1
|
|
-->
|